There exists the dedicated method .str.replace()
for this:
import pandas as pd
# create toy data
df = pd.DataFrame(['9122 15 MHz','9123 15 MHz','9124 15 MHz', '9125 15 MHz','9126 15 MHz','9127 15 MHz','9128 15 MHz','9129 15 MHz','9130 15 MHz','9131 15 MHz'],columns=['col1'])
# replace strings in data
df['col1'].str.replace(' MHz','unit')
In this snippet, I replaced the string ' MHz'
with the term 'unit'
. You can also replace them with an empty string, if you want to get rid of the unit.
Perhaps, you want to extract the unit an convert the column to a numeric value. Do this with (if you have not put your line-number within the toy-example data as I did^^):
df = pd.DataFrame(['15 MHz','15 MHz','15 MHz', '15 MHz','15 MHz','15 MHz','15 MHz','15 MHz','15 MHz','15 MHz'],columns=['col1'])
df['col1'].str.replace(' MHz','').astype('int64')
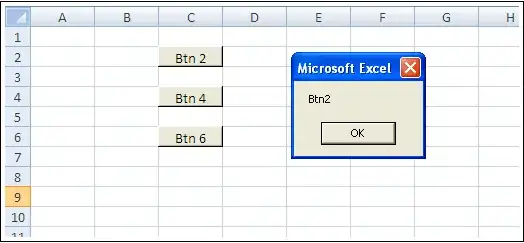
EDIT:
If you have strings of variable length with a variable number of numbers that you want to extract, you can either use re
(regular expression aka. regex) or the build-in string-to-integer function int
. For both, see the answers to this question. I have created an example with both:
import pandas as pd
# create toy data
df = pd.DataFrame(['9122 15 MHz 9123 15 MHz 9124 15 MHz', '9125 15 MHz 9126 15 MHz 9127 15 MHz 9128 15 MHz9129 15 MHz 9130 15 MHz','9131 15 MHz'],columns=['col1'])
# nativ string-to-number conversion:
lst = []
for i,row in df.iterrows():
# get each (positive) number in string
lst.append( [int(s) for s in row['col1'].split() if s.isdigit()] )
# add new list to DataFrame
df['num'] = lst
# regular expression conversion:
import re
lst = []
for i,row in df.iterrows():
lst.append( re.findall(r'\d+', row['col1']) )
# add new list to DataFrame
df['re'] = lst
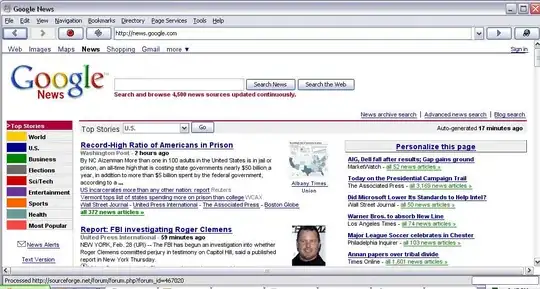