First you need to fix the representation of the maze. The top left of eah cell is self.x + col * 50, self.y + lin * 50
:
class Tabuleiro:
# [...]
def show(self):
for col in range(20):
for lin in range(11):
if self.maze[lin][col] == 1:
self.tela.fill((255, 255, 255), rect=[self.x + col * 50, self.y + lin * 50, 50, 50])
if self.maze[lin][col] == 2:
self.tela.fill((255, 255, 0), rect=[self.x + col * 50 + 12, self.y + lin * 50 + 12, 25, 25])
if self.maze[lin][col] == 3:
self.tela.fill((255, 0, 0), rect=[self.x + col * 50 + 12, self.y + lin * 50 + 12, 25, 25])
You need to add some methods to the Tabuleiro
class. Add a method that can find a specific number
in the grid:
class Tabuleiro:
# [...]
def findFirst(self, number):
for row, rowlist in enumerate(self.maze):
for col, cell in enumerate(rowlist):
if cell == number:
return row, col
return None, None
Add a method that test if a row index (row
) and column index (col
) is valid:
class Tabuleiro:
# [...]
def testIndex(self, row, col):
if row == None or col == None:
return False
if 0 <= row < len(self.maze) and 0 <= col < len(self.maze[row]):
return True
return False
Add a method to test whether the content of a cell is a specific number
:
class Tabuleiro:
# [...]
def isFieldEqual(self, row, col, number):
if self.testIndex(row, col):
return self.maze[row][col] == number
return False
Add a method that swaps the contents of 2 cells:
class Tabuleiro:
# [...]
def swapFileds(self, row1, col1, row2, col2):
if self.testIndex(row1, col1) and self.testIndex(row2, col2):
self.maze[row1][col1], self.maze[row2][col2] = self.maze[row2][col2], self.maze[row1][col1]
There are several methods of detecting keys pressed in Pygame. See How to get if a key is pressed pygame.
For example, use the `KEYDOWN event. Whenever you want to move an object, you need to test that the cell you want to move to is empty (0). When moving, only the contents of the cells are swapped:
while jogo:
row, col = maze.findFirst(2)
for event in pygame.event.get():
if event.type == pygame.QUIT:
jogo = False
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
if maze.isFieldEqual(row, col-1, 0):
maze.swapFileds(row, col-1, row, col)
if event.key == pygame.K_RIGHT:
if maze.isFieldEqual(row, col+1, 0):
maze.swapFileds(row, col+1, row, col)
if event.key == pygame.K_UP:
if maze.isFieldEqual(row-1, col, 0):
maze.swapFileds(row, col, row-1, col)
if event.key == pygame.K_DOWN:
if maze.isFieldEqual(row+1, col, 0):
maze.swapFileds(row+1, col, row, col)
Minimal example:
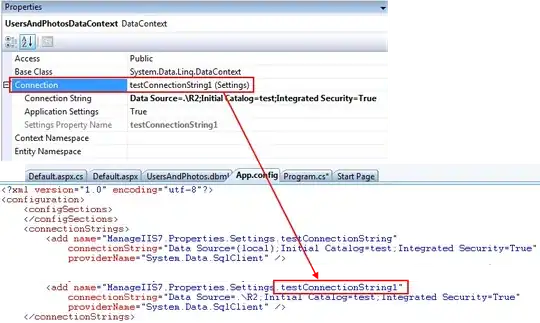
import pygame
pygame.init()
tela = pygame.display.set_mode((1000, 560))
jogo = True
class Tabuleiro:
def __init__(self, tela, x, y):
self.x = x
self.y = y
self.tela = tela
self.maze = [[1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1],
[1, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 1],
[1, 0, 1, 1, 0, 1, 3, 1, 1, 1, 1, 1, 1, 0, 1, 0, 1, 1, 0, 1],
[1, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 3, 1],
[1, 0, 1, 0, 1, 1, 0, 1, 1, 0, 0, 1, 1, 0, 1, 1, 0, 1, 0, 1],
[1, 0, 2, 0, 0, 0, 0, 1, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 1],
[1, 0, 1, 0, 1, 1, 0, 1, 1, 1, 1, 1, 1, 0, 1, 1, 0, 1, 0, 1],
[1, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 1],
[1, 0, 1, 1, 0, 1, 0, 1, 1, 1, 1, 1, 1, 0, 1, 0, 1, 1, 0, 1],
[1, 0, 0, 0, 3, 1, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 1],
[1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1, 1]]
def show(self):
for col in range(20):
for lin in range(11):
if self.maze[lin][col] == 1:
self.tela.fill((255, 255, 255), rect=[self.x + col * 50, self.y + lin * 50, 50, 50])
if self.maze[lin][col] == 2:
self.tela.fill((255, 255, 0), rect=[self.x + col * 50 + 12, self.y + lin * 50 + 12, 25, 25])
if self.maze[lin][col] == 3:
self.tela.fill((255, 0, 0), rect=[self.x + col * 50 + 12, self.y + lin * 50 + 12, 25, 25])
def findFirst(self, number):
for row, rowlist in enumerate(self.maze):
for col, cell in enumerate(rowlist):
if cell == number:
return row, col
return None, None
def testIndex(self, row, col):
if row == None or col == None:
return False
if 0 <= row < len(self.maze) and 0 <= col < len(self.maze[row]):
return True
return False
def isFieldEqual(self, row, col, number):
if self.testIndex(row, col):
return self.maze[row][col] == number
return False
def swapFileds(self, row1, col1, row2, col2):
if self.testIndex(row1, col1) and self.testIndex(row2, col2):
self.maze[row1][col1], self.maze[row2][col2] = self.maze[row2][col2], self.maze[row1][col1]
maze = Tabuleiro(tela, 10, 10)
while jogo:
row, col = maze.findFirst(2)
for event in pygame.event.get():
if event.type == pygame.QUIT:
jogo = False
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
if maze.isFieldEqual(row, col-1, 0):
maze.swapFileds(row, col-1, row, col)
if event.key == pygame.K_RIGHT:
if maze.isFieldEqual(row, col+1, 0):
maze.swapFileds(row, col+1, row, col)
if event.key == pygame.K_UP:
if maze.isFieldEqual(row-1, col, 0):
maze.swapFileds(row, col, row-1, col)
if event.key == pygame.K_DOWN:
if maze.isFieldEqual(row+1, col, 0):
maze.swapFileds(row+1, col, row, col)
tela.fill(0)
maze.show()
pygame.display.update()