I think you've missed to set pytesseract
' page-segmentation-mode (psm
) configuration to 7
which is treating image as a single text line. (source)
I also applied thresholding, my result:
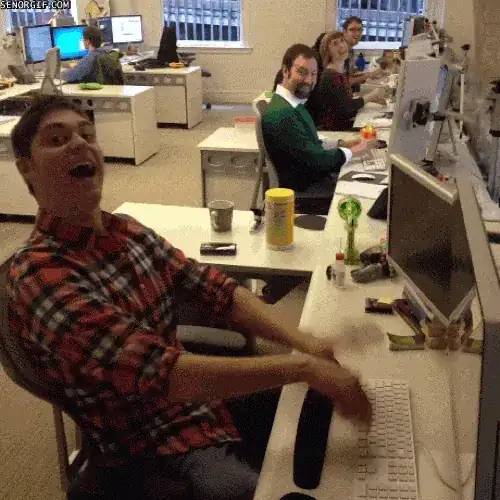
and when I set psm
to 7
txt = pytesseract.image_to_string(thr, config="--psm 7 digits")
print(txt)
Result:
14
Code:
import cv2
import pytesseract
img = cv2.imread("d3njD.jpg")
gry = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
thr = cv2.adaptiveThreshold(gry, 255, cv2.ADAPTIVE_THRESH_MEAN_C,
cv2.THRESH_BINARY_INV, 11, 4)
txt = pytesseract.image_to_string(thr, config="--psm 7 digits")
print(txt)
cv2.imshow("thr", thr)
cv2.waitKey(0)
Please note that, for other images, this solution may not work. You may need additional image processing methods, or you need to change the parameters.
- pytesseract version:
4.1.1