I found this: Graph Visualisation (like yFiles) in JavaFX and changed some code and finaly endet up with this:
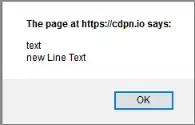
code i changed in the main:
package application;
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Label;
import javafx.scene.control.ScrollPane;
import javafx.scene.layout.AnchorPane;
import javafx.scene.layout.BorderPane;
import javafx.scene.layout.Pane;
import javafx.stage.Stage;
import java.awt.Dimension;
import com.fxgraph.graph.CellType;
import com.fxgraph.graph.Graph;
import com.fxgraph.graph.Model;
import com.fxgraph.layout.base.Layout;
import com.fxgraph.layout.random.RandomLayout;
public class Main extends Application {
Graph graph = new Graph();
@Override
public void start(Stage primaryStage) {
Dimension screenSize = java.awt.Toolkit.getDefaultToolkit().getScreenSize();
double width = screenSize.getWidth();
double height = screenSize.getHeight();
BorderPane root = new BorderPane();
Pane rightPane = new Pane();
rightPane.setPrefWidth(width * 0.4);
rightPane.setPrefHeight(height);
Label infLbl = new Label("Infos");
infLbl.setStyle("-fx-font: 30 arial; -fx-text-fill: red; -fx-font-weight: bold;");
infLbl.setLayoutX(rightPane.getPrefWidth() * 0.1);
infLbl.setLayoutY(rightPane.getPrefHeight() * 0.1);
rightPane.getChildren().add(infLbl);
graph = new Graph();
ScrollPane scroll = graph.getScrollPane();
// scroll.setMaxWidth(width - width * 0.3);
root.setCenter(scroll);
root.setRight(rightPane);
Scene scene = new Scene(root);
scene.getStylesheets().add(getClass().getResource("application.css").toExternalForm());
primaryStage.setWidth(width);
primaryStage.setHeight(height);
primaryStage.setScene(scene);
primaryStage.show();
addGraphComponents();
Layout layout = new RandomLayout(graph);
layout.execute();
}
private void addGraphComponents() {
Model model = graph.getModel();
graph.beginUpdate();
model.addCell("Cell A", CellType.RECTANGLE);
model.addCell("Cell B", CellType.RECTANGLE);
model.addCell("Cell C", CellType.RECTANGLE);
model.addCell("Cell D", CellType.TRIANGLE);
model.addCell("Cell E", CellType.TRIANGLE);
model.addCell("Cell F", CellType.RECTANGLE);
model.addCell("Cell G", CellType.RECTANGLE);
model.addEdge("Cell A", "Cell B");
model.addEdge("Cell A", "Cell C");
model.addEdge("Cell B", "Cell C");
model.addEdge("Cell C", "Cell D");
model.addEdge("Cell B", "Cell E");
model.addEdge("Cell D", "Cell F");
model.addEdge("Cell D", "Cell G");
graph.endUpdate();
}
public static void main(String[] args) {
launch(args);
}
}
and some changes in the RectangleCell class:
package com.fxgraph.cells;
import javafx.scene.control.Label;
import javafx.scene.layout.StackPane;
import javafx.scene.paint.Color;
import javafx.scene.shape.Rectangle;
import com.fxgraph.graph.Cell;
public class RectangleCell extends Cell {
public RectangleCell( String id) {
super( id);
Rectangle view = new Rectangle( 50,50);
view.setStroke(Color.DODGERBLUE);
view.setFill(Color.DODGERBLUE);
Label lbl = new Label(id);
//lbl.setClip(view);
lbl.setLabelFor(view);
StackPane stack = new StackPane();
stack.getChildren().addAll(view, lbl);
setView(stack);
}
}
maybe there is a way to add an hierarchical layout to this and can save a spot with id for the rectangle so that it could look like this (ids not shown in the final application):
