Rearrange data in row slices and write to ListObject
Apparently OP doesn't want only a simple unpivot action, but to rearrange row values and (over)write a given ListObject. The following code demonstrates this benefitting
Sub Rearrange(rng As Range)
'[0] get data
Dim data: data = rng
Dim categories: categories = Application.Index(data, 1, 0)
'[1] provide for sufficient array rows
Dim cnt As Long: cnt = UBound(data)
Dim results: ReDim results(1 To (UBound(categories) - 1) * (cnt - 1))
'~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
'[2] arrange data in wanted order
'~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
Dim cat As Long
For cat = 2 To UBound(categories) ' Height, Weight, Color
Dim i As Long, ii As Long
For i = 2 To cnt ' e.g. data rows 2:4
' collect all relevant data in row columns and
' overwrite inserted 2nd col with category
Dim currData
currData = Application.Index(data, Evaluate("row(" & i & ":" & i & ")"), Array(1, 0, cat))
currData(2) = categories(cat) ' replace 2nd column w/ category
'
ii = ii + 1: results(ii) = currData ' increment row counter
Next i
Next cat
'put array rows together
results = Application.Index(results, 0, 0)
'[3] write results to target
' a) define captions
Dim captions: captions = Split("Code,Ref,Value", ",")
' b) write to another listobject or overwrite given listobject
' (~~> change target to your needs)
With Tabelle1.ListObjects("MyTable2")
' Get number of rows to adjust
Dim rowCorr As Long, colCorr As Long
rowCorr = UBound(results) - LBound(results) + 1 - .DataBodyRange.Rows.Count
colCorr = UBound(results, 2) - LBound(results, 2) + 1 - .DataBodyRange.Columns.Count
Debug.Print "Rows/Cols to adjust = " & rowCorr & "/" & colCorr
'Adjust list object
If rowCorr < 0 Then ' Delete Rows
.DataBodyRange.Rows(1).Resize(Abs(rowCorr)).Delete xlShiftUp
ElseIf rowCorr > 0 Then ' Insert rows
.DataBodyRange.Rows(1).Resize(rowCorr).Insert Shift:=xlDown
End If
If colCorr < 0 Then ' Delete Cols
.Range.Resize(, Abs(colCorr)).Columns.Delete
ElseIf colCorr > 0 Then ' Insert cols
.Range.Resize(, colCorr).Columns.Insert
End If
'overwrite data
.HeaderRowRange = captions
.DataBodyRange = results
End With
End Sub
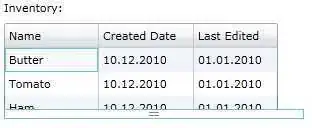
Calling code example
You could start the wanted rearrangements e.g. by
Rearrange Sheet1.ListObjects("MyTable").Range
or even with
Rearrange Sheet1.Range("A1:D3")
If you might want to write to a range target (of another sheet for instance) instead of a ListObject, you could replace section [3]b)
e.g. with
With Sheet2.Range("A1")
.Resize(1, UBound(captions) + 1) = captions
.Offset(1).Resize(UBound(results), UBound(results, 2)) = results
End With
and/or split the code into several sub procedures.