Formula for cubic Bezier curve component (say X):
X(t) = P0.X*(1-t)^3 + 3*P1.X*(1-t)^2*t + 3*P2.X*(1-t)*t^2 + P3.X*t^3
where P0
and P3
are end points, and P1
an P2
are control points.
We have four points for curve (SrcPt
array in that answer), end points coincide with Bezier endpoints, and two internal points on curve should define two control points P1
an P2
of Bezier curve. To calculate, we must know - what t
parameters correspond to SrcPt[1]
and SrcPt[2]
. Let these parameters are 1/3 and 2/3 (possible issues are in linked answers).
So substitute t=1/3
and t=2/3
into the formula above:
SrcPt[1].X = SrcPt[0].X*(1-1/3)^3 + 3*P1.X*(1-1/3)^2*1/3 +
3*P2.X*(1-1/3)*1/3^2 + SrcPt[3].X*1/3^3
SrcPt[2].X = SrcPt[0].X*(1-2/3)^3 + 3*P1.X*(1-2/3)^2*2/3 +
3*P2.X*(1-2/3)*(2/3)^2 + SrcPt[3].X*2/3)^3
and solve this system for unknown P1.X
and P2.X
. So we will have all needed points to describe Bezier curve. Linked answer implements the solution.
Example - how changing t
values for the same internal points influences on curve:
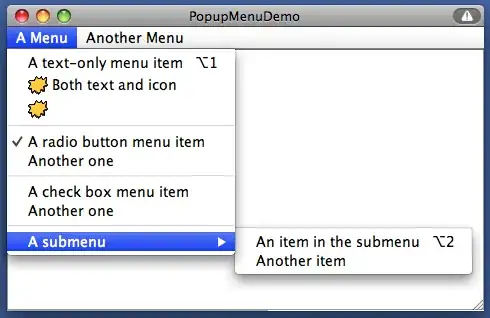