After install 'Microsoft.Office.Interop.Outlook' nuget package, you can try the following code to send email (code from https://stackoverflow.com/a/42743804/12666543):
Imports System.IO
Imports Outlook = Microsoft.Office.Interop.Outlook
Module Module1
Sub Main()
Dim arrAttachFiles As List(Of String) = New List(Of String)() From {
"the file path you want to attach (e.g. D:\Test.xlsx)"
}
sendEmailViaOutlook("your email address", "email addresses you want to send", "email addresses you want to cc", "this is subject", "this is body", arrAttachFiles)
End Sub
Public Sub sendEmailViaOutlook(ByVal sFromAddress As String, ByVal sToAddress As String, ByVal sCc As String, ByVal sSubject As String, ByVal sBody As String, ByVal Optional arrAttachments As List(Of String) = Nothing)
Try
Dim app As Outlook.Application = New Outlook.Application()
Dim newMail As Outlook.MailItem = CType(app.CreateItem(Outlook.OlItemType.olMailItem), Outlook.MailItem)
If Not String.IsNullOrWhiteSpace(sToAddress) Then
Dim arrAddTos As String() = sToAddress.Split(New Char() {";"c, ","c})
For Each strAddr As String In arrAddTos
If Not String.IsNullOrWhiteSpace(strAddr) AndAlso strAddr.IndexOf("@"c) <> -1 Then
newMail.Recipients.Add(strAddr.Trim())
Else
Throw New Exception("Bad to-address: " & sToAddress)
End If
Next
Else
Throw New Exception("Must specify to-address")
End If
If Not String.IsNullOrWhiteSpace(sCc) Then
Dim arrAddTos As String() = sCc.Split(New Char() {";"c, ","c})
For Each strAddr As String In arrAddTos
If Not String.IsNullOrWhiteSpace(strAddr) AndAlso strAddr.IndexOf("@"c) <> -1 Then
newMail.Recipients.Add(strAddr.Trim())
Else
Throw New Exception("Bad CC-address: " & sCc)
End If
Next
End If
If Not newMail.Recipients.ResolveAll() Then
Throw New Exception("Failed to resolve all recipients: " & sToAddress & ";" & sCc)
End If
If arrAttachments IsNot Nothing Then
For Each strPath As String In arrAttachments
If File.Exists(strPath) Then
newMail.Attachments.Add(strPath)
Else
Throw New Exception("Attachment file is not found: """ & strPath & """")
End If
Next
End If
If Not String.IsNullOrWhiteSpace(sSubject) Then newMail.Subject = sSubject
If Not String.IsNullOrWhiteSpace(sBody) Then newMail.Body = sBody
Dim accounts As Outlook.Accounts = app.Session.Accounts
Dim acc As Outlook.Account = Nothing
For Each account As Outlook.Account In accounts
If account.SmtpAddress.Equals(sFromAddress, StringComparison.CurrentCultureIgnoreCase) Then
acc = account
Exit For
End If
Next
If acc IsNot Nothing Then
newMail.SendUsingAccount = acc
newMail.Send()
Else
Throw New Exception("Account does not exist in Outlook: " & sFromAddress)
End If
Catch ex As Exception
Console.WriteLine("ERROR: Failed to send mail: " & ex.Message)
End Try
End Sub
End Module
Result:
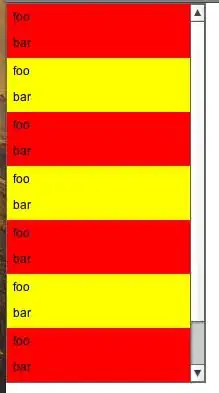