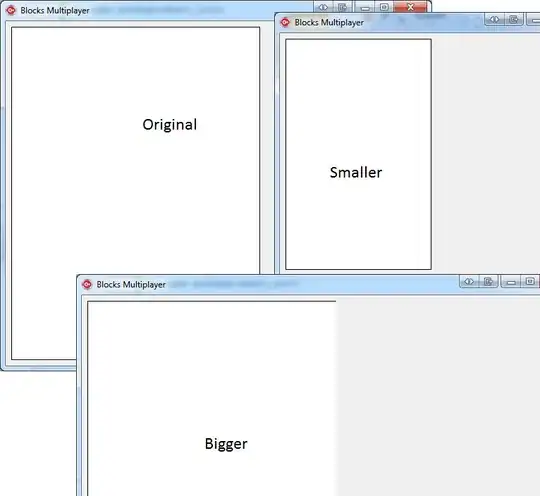
I created a minimally reproduceable model of the clipping plane. From DevDept's Volumetric Rendering Example. Two things I noticed are that you need to ensure the viewport is redrawn (viewport.Invalidate(); ) after the Edit(null) is called. Also in the example Plane.Origin.Z is messed with. Somehow this does not get re-rendered unless the full plane of the clippingPlane1 is changed:
viewportLayout1.ClippingPlane1.Plane = new Plane(new Point3D(7, 7, 7), new Vector3D(0, 0, 1));
Code for ClippingPlane1:
private void addClippingPlane()
{
// Remove Clipping Plane if Active
if (viewportLayout1.ClippingPlane1.Active) { viewportLayout1.ClippingPlane1.Cancel(); }
//sets the Z coordinate of the origin of the clippingPlane
viewportLayout1.ClippingPlane1.Plane = new Plane(new Point3D(7, 7, 7), new Vector3D(0, 0, 1));
// enables a clippingPlane
viewportLayout1.ClippingPlane1.Edit(null);
// refresh the viewport
viewportLayout1.Invalidate();
}
Another method to clip meshes is CutBy. This method allows meshes to be cut individually by a plane. Unfortunately plane is a Geometry child vice and Entity Child, so it cannot be added to the viewport as a plane. To alleviate this, it can be drawn using the Quad entity, with a length given for the drawn sides.
CutBy Example:
private void addCutByPlane()
{
// Remove Clipping Plane if Active
if (viewportLayout1.ClippingPlane1.Active) { viewportLayout1.ClippingPlane1.Cancel(); }
// Create Meshes
Plane cutPlane1 = new Plane(new Point3D(7, 7, 7), new Vector3D(0, 0, 1));
Mesh square = Mesh.CreateBox(15, 15, 15);
// Cut Mesh by Plane
square.CutBy(cutPlane1);
// Length of Drawn part of plane
double drawPlaneLength = 16;
double hL = drawPlaneLength / 2;
// Draw Plane region as a Quad Entity
Point3D pO = cutPlane1.Origin;
Point3D q1 = (pO + (cutPlane1.AxisX + cutPlane1.AxisY) * hL);
Point3D q2 = (pO - (cutPlane1.AxisX - cutPlane1.AxisY) * hL);
Point3D q3 = (pO - (cutPlane1.AxisX + cutPlane1.AxisY) * hL);
Point3D q4 = (pO + (cutPlane1.AxisX - cutPlane1.AxisY) * hL);
Quad drawCutPlane1 = new Quad(q1, q2, q3, q4);
viewportLayout1.Entities.Clear();
viewportLayout1.Entities.Add(drawCutPlane1, Color.Red);
viewportLayout1.Entities.Add(square, Color.Blue);
viewportLayout1.Invalidate();
}
Full Example:
using System;
using System.Drawing;
using System.Windows.Forms;
using devDept.Eyeshot.Entities;
using devDept.Geometry;
namespace EyeshotTest
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
makeSquare();
}
private void makeSquare()
{
Mesh square = Mesh.CreateBox(15, 15, 15);
viewportLayout1.Entities.Add(square, Color.Green);
viewportLayout1.Invalidate();
}
private void addClippingPlane()
{
// Remove Clipping Plane if Active
if (viewportLayout1.ClippingPlane1.Active) { viewportLayout1.ClippingPlane1.Cancel(); }
//sets the Z coordinate of the origin of the clippingPlane
viewportLayout1.ClippingPlane1.Plane = new Plane(new Point3D(7, 7, 7), new Vector3D(0, 0, 1));
// enables a clippingPlane
viewportLayout1.ClippingPlane1.Edit(null);
// refresh the viewport
//viewportLayout1.Entities.Regen();
// ^ unneeded, but when in doubt always Regen & Invalidate
// Then remove them where ever possible for speed improvments
viewportLayout1.Invalidate();
}
private void button1_Click(object sender, EventArgs e)
{
addClippingPlane();
}
private void addCutByPlane()
{
// Remove Clipping Plane if Active
if (viewportLayout1.ClippingPlane1.Active) { viewportLayout1.ClippingPlane1.Cancel(); }
// Create Meshes
Plane cutPlane1 = new Plane(new Point3D(7, 7, 7), new Vector3D(0, 0, 1));
Mesh square = Mesh.CreateBox(15, 15, 15);
// Cut Mesh by Plane
square.CutBy(cutPlane1);
// Length of Drawn part of plane
double drawPlaneLength = 16;
double hL = drawPlaneLength / 2;
// Draw Plane region as a Quad Entity
Point3D pO = cutPlane1.Origin;
Point3D q1 = (pO + (cutPlane1.AxisX + cutPlane1.AxisY) * hL);
Point3D q2 = (pO - (cutPlane1.AxisX - cutPlane1.AxisY) * hL);
Point3D q3 = (pO - (cutPlane1.AxisX + cutPlane1.AxisY) * hL);
Point3D q4 = (pO + (cutPlane1.AxisX - cutPlane1.AxisY) * hL);
Quad drawCutPlane1 = new Quad(q1, q2, q3, q4);
viewportLayout1.Entities.Clear();
viewportLayout1.Entities.Add(drawCutPlane1, Color.Red);
viewportLayout1.Entities.Add(square, Color.Blue);
viewportLayout1.Invalidate();
}
private void button2_Click(object sender, EventArgs e)
{
addCutByPlane();
}
}
}