From a broader perspective there is nothing wrong in your code.
However to optimize your code you may like to:
Induce WebDriverWait for the element_to_be_clickable()
for the expander to be clickable.
The texts EU Odds, UK Odds, US Odds, etc are not exactly within the <li>
but within it's grand parent //li/a/span
So to select EU Odds, UK Odds, US Odds one by one your optimized Locator Strategies can be:
driver.get('https://www.oddsportal.com/matches/tennis/')
WebDriverWait(driver, 20).until(EC.element_to_be_clickable((By.ID, "user-header-oddsformat-expander"))).click()
target = "EU Odds"
WebDriverWait(driver, 20).until(EC.element_to_be_clickable((By.XPATH, "//li/a/span[text()='" + target + "']"))).click()
time.sleep(3) # for visual demonstration
WebDriverWait(driver, 20).until(EC.element_to_be_clickable((By.ID, "user-header-oddsformat-expander"))).click()
target = "UK Odds"
WebDriverWait(driver, 20).until(EC.element_to_be_clickable((By.XPATH, "//li/a/span[text()='" + target + "']"))).click()
time.sleep(3) # for visual demonstration
WebDriverWait(driver, 20).until(EC.element_to_be_clickable((By.ID, "user-header-oddsformat-expander"))).click()
target = "US Odds"
WebDriverWait(driver, 20).until(EC.element_to_be_clickable((By.XPATH, "//li/a/span[text()='" + target + "']"))).click()
This usecase
However even sucessfully clicking on the options with text as EU Odds, UK Odds, US Odds doesn't changes the DOM Tree. Now if you look into the HTML DOM the expander class itself is having the value as user-header-fakeselect-options
. Additionally, the onclick
attribute of the //li/a/span
items are having return false;
.
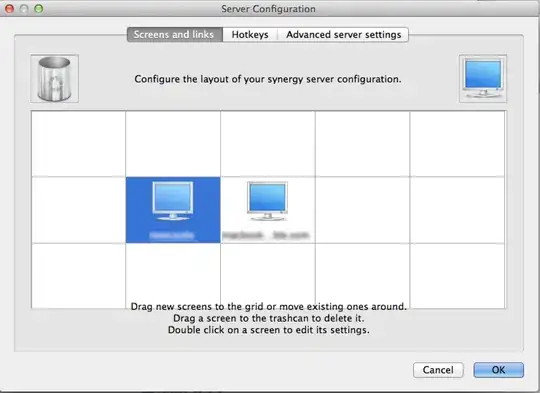
As per the discussion What's the effect of adding 'return false' to a click event listener? return false;
does three separate things when called:
- Calls
event.preventDefault();
- Calls
event.stopPropagation();
- Stops callback execution and returns immediately when called.
Hence selecting either of the dropdown options doesn't fires the onclick
event and the HTML DOM remains unchanged.
Conclusion
Possibly the DOM would change as per the onclick
events when a authenticated user performs the selection after loggind in within the website.