You could try the code below.
layout_SwitchView.xml:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="match_parent"
android:layout_height="match_parent">
<include
android:id="@+id/FirstLayout"
layout="@layout/layout_sw_first"
android:layout_width="match_parent"
android:layout_height="wrap_content"/>
<include
android:id="@+id/SecondLayout"
layout="@layout/layout_sw_second"
android:visibility="gone"
android:layout_width="match_parent"
android:layout_height="wrap_content"/>
<include
android:id="@+id/menu_bar"
layout="@layout/layout_sw_menubar"
android:layout_width="match_parent"
android:layout_height="wrap_content"/>
</LinearLayout>
layout_SW_first.xml:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView
android:text="First Layout"
android:layout_width="match_parent"
android:layout_height="wrap_content"/>
</LinearLayout>
layout_SW_second.xml:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="match_parent"
android:layout_height="match_parent">
layout_SW_menubar.xml:
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical"
android:layout_width="match_parent"
android:layout_height="match_parent">
<LinearLayout
android:orientation="horizontal"
android:layout_width="fill_parent"
android:layout_height="75dp"
android:weightSum="100"
android:layout_alignParentBottom="true">
<LinearLayout
android:orientation="vertical"
android:layout_weight="20"
android:layout_width="0dp"
android:layout_height="match_parent"
android:id="@+id/FirstMenuItem"
android:paddingTop="6dp"
android:clickable="true"
android:focusable="true"
android:focusableInTouchMode="true">
<ImageView
android:src="@drawable/tab_graph"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/imageView1"
android:scaleType="fitCenter"
android:adjustViewBounds="false" />
<TextView
android:text="First"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/textView1"
android:gravity="center_horizontal" />
</LinearLayout>
<LinearLayout
android:orientation="vertical"
android:layout_weight="20"
android:layout_width="0dp"
android:layout_height="match_parent"
android:id="@+id/SecondMenuItem"
android:paddingTop="6dp"
android:clickable="true"
android:focusable="true"
android:focusableInTouchMode="true">
<ImageView
android:src="@drawable/tab_chat"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/imageView2"
android:scaleType="fitCenter"
android:adjustViewBounds="false" />
<TextView
android:text="Second"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/textView2"
android:gravity="center_horizontal" />
</LinearLayout>
<LinearLayout
android:orientation="vertical"
android:layout_weight="20"
android:layout_width="0dp"
android:layout_height="match_parent"
android:id="@+id/moreMenuItem"
android:paddingTop="6dp"
android:clickable="true"
android:focusable="true"
android:focusableInTouchMode="true">
<ImageView
android:src="@drawable/star_small"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/imageView5"
android:scaleType="fitCenter"
android:adjustViewBounds="false" />
<TextView
android:text="More"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/textView5"
android:gravity="center_horizontal" />
</LinearLayout>
</LinearLayout>
</RelativeLayout>
Activity_SwitchView.cs:
protected override void OnCreate(Bundle savedInstanceState)
{
base.OnCreate(savedInstanceState);
// Create your application here
SetContentView(Resource.Layout.layout_SwitchView);
LinearLayout FirstMenuItemLayout = FindViewById<LinearLayout>(Resource.Id.FirstMenuItem);
LinearLayout SecondMenuItemLayout = FindViewById<LinearLayout>(Resource.Id.SecondMenuItem);
LinearLayout firstlayout = FindViewById<LinearLayout>(Resource.Id.FirstLayout);
LinearLayout secondlayout = FindViewById<LinearLayout>(Resource.Id.SecondLayout);
FirstMenuItemLayout.Click += (sender, args) =>
{
firstlayout.Visibility = ViewStates.Visible;
secondlayout.Visibility = ViewStates.Gone;
};
SecondMenuItemLayout.Click += (sender, args) =>
{
firstlayout.Visibility = ViewStates.Gone;
secondlayout.Visibility = ViewStates.Visible;
};
}
Screenshot:
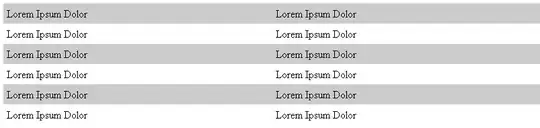