I understand that your firestore collection is similar to this one:
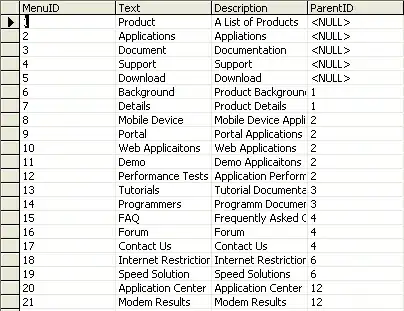
If that is the case, then I have structured your requirement in the three functions below:
1.readBlockedNumbers
to return the array of blocked numbers that the user has.
2.show_nonBlockedUsers
that receives the array of the blocked numbers from the previous method and displays the users who are not in this array.
3. test
to coordinate the execution of the above two methods.
const admin = require('firebase-admin');
const serviceAccount = require('/home/keys.json');
admin.initializeApp({
credential: admin.credential.cert(serviceAccount)
});
const db = admin.firestore();
async function readBlockedNumbers(docId){
const userRef = db.collection('users').doc(docId);
const doc = await userRef.get();
if (!doc.exists) {
console.log('No such document!');
return [];
}
else
{
return doc.data()['blocked_numbers'];
}
async function show_nonBlockedUsers(blocked_users){
console.log('Array length:', blocked_users.length);
if(blocked_users.length == 0)
return;
const userRef = db.collection('users');
const snapshot = await userRef
.where(admin.firestore.FieldPath.documentId(), 'not-in', blocked_users)
.get();
if (snapshot.empty) {
console.log('No matching documents.');
return;
}
snapshot.forEach(doc => {
console.log(doc.id, '=>', doc.data());
});
}
async function test(){
const docId = '202-555-0146';
//'202-555-0102'
const blocked_users = await readBlockedNumbers(docId);
await show_nonBlockedUsers(blocked_users);
}
Important here is how to use the not-in
operator and the method admin.firestore.FieldPath.documentId().
I have found the not-in
operator here and the method firebase.firestore.FieldPath.documentId()
referenced in this other stackoverflow question since the id
cannot be passed like the other document fields in the where clause.
Please refer to the firebase documentation as well for the limitations of the not-in
operator.
I hope you find this useful.