The java.util.Date
object is not a real date-time object like the modern date-time types; rather, it represents the number of milliseconds since the standard base time known as "the epoch", namely January 1, 1970, 00:00:00 GMT
(or UTC). When you print an object of java.util.Date
, its toString
method returns the date-time in the JVM's timezone, calculated from this milliseconds value. If you need to print the date-time in a different timezone, you will need to set the timezone to SimpleDateFormat
and obtain the formatted string from it.
Note that the date-time API of java.util
and their formatting API, SimpleDateFormat
are outdated and error-prone. It is recommended to stop using them completely and switch to the modern date-time API.
Using the modern date-time API:
import java.time.Instant;
import java.time.LocalDate;
import java.time.LocalDateTime;
import java.time.LocalTime;
import java.time.Month;
import java.time.ZoneId;
import java.time.ZoneOffset;
import java.time.ZonedDateTime;
class Appointment {
private LocalDateTime appointmentStartDatetime;
private String timezone;
public Appointment(LocalDateTime appointmentStartDatetime, String timezone) {
this.appointmentStartDatetime = appointmentStartDatetime;
this.timezone = timezone;
}
public LocalDateTime getAppointmentInLocalDateTime() {
return appointmentStartDatetime;
}
public Instant getAppointmentInUTC() {
return appointmentStartDatetime.toInstant(ZoneOffset.UTC);
}
public ZonedDateTime getAppointmentInZonedDateTime() {
return appointmentStartDatetime.atZone(ZoneId.of(timezone));
}
public ZonedDateTime getAppointmentInZdtAnotherTimeZone(String timezone) {
return getAppointmentInZonedDateTime().withZoneSameInstant(ZoneId.of(timezone));
}
// ...
}
public class Main {
public static void main(String[] args) {
Appointment appointment = new Appointment(
LocalDateTime.of(LocalDate.of(2020, Month.FEBRUARY, 15), LocalTime.of(10, 30)), "America/New_York");
System.out.println(appointment.getAppointmentInLocalDateTime());
System.out.println(appointment.getAppointmentInUTC());
System.out.println(appointment.getAppointmentInZonedDateTime());
System.out.println(appointment.getAppointmentInZdtAnotherTimeZone("Asia/Calcutta"));
}
}
Output:
2020-02-15T10:30
2020-02-15T10:30:00Z
2020-02-15T10:30-05:00[America/New_York]
2020-02-15T21:00+05:30[Asia/Calcutta]
- An
Instant
represents an instantaneous point on the time-line.
- A
ZonedDateTime
holds state equivalent to three separate objects, a LocalDateTime
, a ZoneId
and the resolved ZoneOffset
. The function, ZonedDateTime#withZoneSameInstant
returns a copy of this date-time with a different time-zone, retaining the instant.
Given below is an overview of the Java SE 8 date-time types:
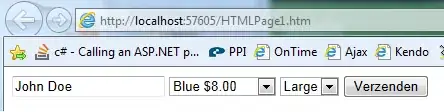
Learn more about the modern date-time API from Trail: Date Time.