This is one way of animating a car image. The OP didn't provide car images, so I created one.
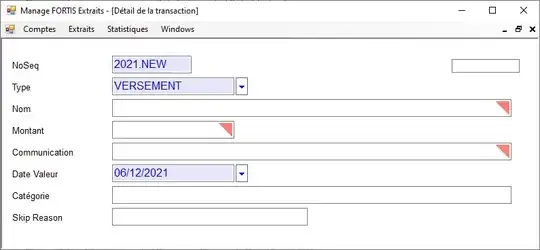
Here's the GUI I created. The car moves from right to left.

I use the model / view / controller pattern when I create a Swing application. This model enforces a separation of concerns and allows me to focus on one part of the Swing application at a time.
So, the first thing I did was to create a Car
class. The Car
class holds the position of the image and the image itself.
Generally, in a Swing application, you read all the resources first, then you create your GUI. If reading the resources takes a long time, you can read the resources in a separate Thread that will run while you build the GUI.
Since I had one resource, I read it.
After I created the Car
class, I created a view with one JFrame
and one drawing JPanel
. Generally, you create one drawing JPanel
and draw everything on that one drawing JPanel
.
The controller in this application is the Swing Timer
. The Timer
moves the car from right to left and starts over when the car reaches the left edge of the drawing JPanel
.
Here's the complete runnable code.
import java.awt.BorderLayout;
import java.awt.Color;
import java.awt.Dimension;
import java.awt.Graphics;
import java.awt.Point;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.image.BufferedImage;
import java.io.IOException;
import javax.imageio.ImageIO;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.SwingUtilities;
import javax.swing.Timer;
public class CarAnimationGUI implements Runnable {
public static void main(String[] args) {
SwingUtilities.invokeLater(new CarAnimationGUI());
}
private int drawingAreaWidth;
private int drawingAreaHeight;
private int startX, startY;
private Car car;
private DrawingPanel drawingPanel;
public CarAnimationGUI() {
this.drawingAreaWidth = 600;
this.drawingAreaHeight = 200;
this.car = new Car();
BufferedImage image = car.getImage();
this.startX = drawingAreaWidth - image.getWidth();
this.startY = drawingAreaHeight - image.getHeight();
car.setLocation(new Point(startX, startY));
}
@Override
public void run() {
JFrame frame = new JFrame("Car Animation GUI");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
this.drawingPanel = new DrawingPanel(drawingAreaWidth,
drawingAreaHeight);
frame.add(drawingPanel, BorderLayout.CENTER);
frame.pack();
frame.setLocationByPlatform(true);
frame.setVisible(true);
Timer animate = new Timer(50, new CarMover(startX, startY));
animate.start();
}
public void repaint() {
drawingPanel.repaint();
}
public class DrawingPanel extends JPanel {
private static final long serialVersionUID = 1L;
public DrawingPanel(int width, int height) {
this.setBackground(new Color(154, 232, 208));
this.setPreferredSize(new Dimension(width, height));
}
@Override
protected void paintComponent(Graphics g) {
super.paintComponent(g);
BufferedImage image = car.getImage();
Point point = car.getLocation();
g.drawImage(image, point.x, point.y, this);
}
}
private class CarMover implements ActionListener {
private final int startX, startY;
private int x;
public CarMover(int startX, int startY) {
this.startX = startX;
this.startY = startY;
}
@Override
public void actionPerformed(ActionEvent event) {
x -= 3;
x = (x < 0) ? startX : x;
car.setLocation(new Point(x, startY));
repaint();
}
}
public class Car {
private final BufferedImage image;
private Point location;
public Car() {
this.image = setImage();
}
private BufferedImage setImage() {
try {
return ImageIO.read(getClass()
.getResourceAsStream("/car.jpg"));
} catch (IOException e) {
e.printStackTrace();
return null;
}
}
public BufferedImage getImage() {
return image;
}
public void setLocation(Point location) {
this.location = location;
}
public Point getLocation() {
return location;
}
}
}