The problem you're running into is that Spree::BaseController
already inherits from ApplicationController
; see https://github.com/spree/spree/blob/master/core/app/controllers/spree/base_controller.rb. This is to allow your ApplicationController
to define things like current_user
and similar basic functions before Spree sees it.
Declaring them the other way around as well creates a circular dependency, and the class loading fails as a result. Without changing Spree itself, the only fix is to do something else.
Instead, to have your controllers use Spree::BaseController
as a superclass, first define ApplicationController
in the more usual fashion e.g.:
# app/controllers/application_controller.rb
class ApplicationController < ActionController::Base
# ...
end
then invent a new abstract controller, for your own use, that inherits from Spree, e.g. let's name it StoreBaseController
:
# app/controllers/store_base_controller.rb
class StoreBaseController < Spree::BaseController
include Spree::Core::ControllerHelpers::Order
# ...
end
This StoreBaseController
can now be used in place of ApplicationController
when defining more specific controllers. It works because it doesn't create a loop in the inheritance tree, which now looks like this:
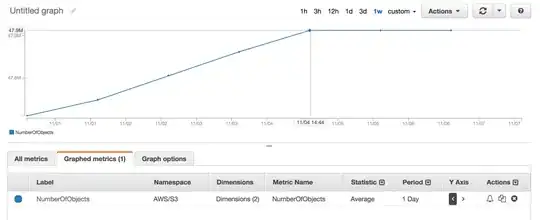
Note: if you're also using the rails generator
command to produce controllers or scaffolds from templates, be aware that the generator has ApplicationController
hard-coded in the templates, so you'll need to amend them once created.