With flatten()
you can get the name and values of every key inside a json:
with variants as (
select 1 id, parse_json('{"d1":1, "d2":2, "d3":3}') data
union all select 2, parse_json('{"d1":4, "d2":5, "d3":7}')
union all select 3, parse_json('{"d1":2, "d2":0, "d3":0}')
)
select *
from variants, table(flatten(data));
;
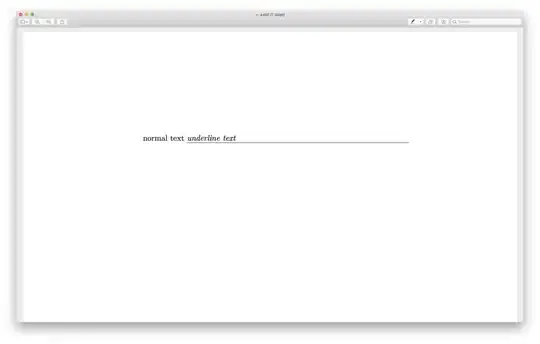
Given that information, you can look into all the keys named like d%
and look for a value that doesn't exist in any - let's say 2:
with variants as (
select 1 id, parse_json('{"d1":1, "d2":2, "d3":3}') data
union all select 2, parse_json('{"d1":4, "d2":5, "d3":7}')
union all select 3, parse_json('{"d1":2, "d2":0, "d3":0}')
)
select id, not boolor_agg((iff(key like 'd%', value=2, true))) doesnt_have_a_2
from variants, table(flatten(data))
group by id
;
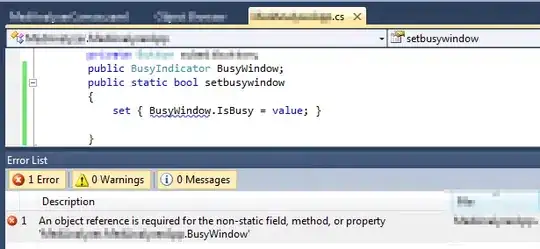
That shows you that the row with id=2
is the only row where no key has the value 2
.
As an alternative, you could also filter the key names in the where
clause instead of iff
:
with variants as (
select 1 id, parse_json('{"d1":1, "d2":2, "d3":3}') data
union all select 2, parse_json('{"d1":4, "d2":5, "d3":7}')
union all select 3, parse_json('{"d1":2, "d2":0, "d3":0}')
)
select id, boolor_agg(value=2)
from variants, table(flatten(data))
where key like 'd%'
group by id
;