I would suggest using a different toolbox to add blur to images but in theory it would be possible using matplotlib.
For example
import matplotlib.pyplot as plt
fig, ax = plt.subplots()
def add_blur(center = (0, 0), n = 10, radius = .5, blur = 1.1):
artists = [plt.Circle(center, radius = radius)]
for i in range(0, n):
r = (n - i)/ (n) * ( blur * radius)
alpha = i/(n)
a = plt.Circle(center, radius = r, alpha = alpha)
artists.append(a)
return artists
ns = [200, 1]
centers = [(-1, 0), (1, 0)]
for center, n in zip(centers, ns):
artists = add_blur(center = center, n = n)
[ax.add_artist(a) for a in artists]
ax.axis('equal')
ax.set(xlim = (-2, 2), ylim = (-2 ,2),
)
fig.show()
Gives:
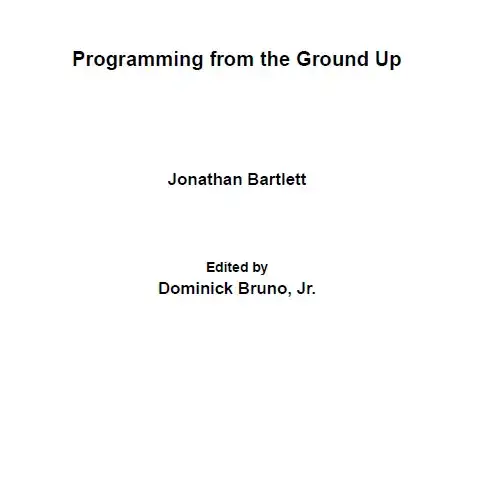