Remember to assign Application permission (Client credentials flow) to the app registration. See Application permission to Microsoft Graph.
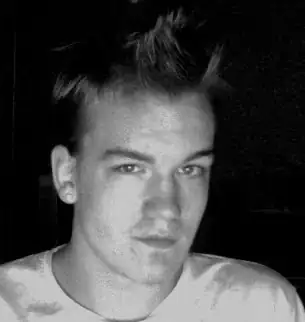
You can use Client credentials provider.
IConfidentialClientApplication confidentialClientApplication = ConfidentialClientApplicationBuilder
.Create(clientId)
.WithTenantId(tenantID)
.WithClientSecret(clientSecret)
.Build();
ClientCredentialProvider authProvider = new ClientCredentialProvider(confidentialClientApplication);
Upload small file (<4M):
GraphServiceClient graphClient = new GraphServiceClient(authProvider);
using var stream = new System.IO.MemoryStream(Encoding.UTF8.GetBytes("The contents of the file goes here."));
await graphClient.Users[{upn or userID}].Drive.Items["{item-id}"].Content
.Request()
.PutAsync<DriveItem>(stream);
If you want to upload large file, see Upload large files with an upload session.
UPDATE:
Use Install-Package Microsoft.Graph.Auth -IncludePrerelease
in Tools -> NuGet Package Manager -> Package Manager Console.
It supports both uploading a new file and replacing an existing item.
For how to get the items id, please refer to Get a file or folder.
For example, get the item id of /folder1/folder2
(have a quick test in Microsoft Graph Explorer):
GET https://graph.microsoft.com/v1.0/users/{userID}/drive/root:/folder1:/children
It will list all the children in folder1, including folder2. Then you can find item id of folder2.
UPDATE 2:
Client credentials provider is application identity while all the providers below are user identity.
Since you want to access personal OneDrive (including user identity), you could choose Authorization code provider or Interactive provider.
Authorization code provider: you need to implement interactively sign-in for your web app and use this provider.
Interactive provider: you can easily use this provider to implement interactively sign-in in a console app.
You can have a quick test with the second provider.
Please note that you should add the Delegated (personal Microsoft account) permissions into the app registration. See Delegated permission to Microsoft Graph.
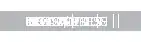
And in this case, you should modify all graphClient.Users[{upn or userID}]
to graphClient.Me
in your code.
I'm afraid that you have to implement sign-in interactively auth flow to access personal OneDrive.