I have created a horizontal scrolling collection view within a parent UIView, which I add in my UIViewController. But the scrolling view shifts up when I scroll on it.
This is how the container view is initialized:
override init(frame: CGRect){
let layout = UICollectionViewFlowLayout()
layout.scrollDirection = .horizontal
layout.itemSize = UICollectionViewFlowLayoutAutomaticSize
layout.estimatedItemSize = CGSize(width: 100, height: 50)
countryCollectionView = UICollectionView(frame: CGRect.zero, collectionViewLayout: layout)
viewTitleLabel = UILabel()
super.init(frame: frame)
setupTableView()
sortedDummyData = dummyData.sorted(by: <)
}
Constraints on my child views:
NSLayoutConstraint.activate([
viewTitleLabel.leadingAnchor.constraint(equalTo: self.leadingAnchor, constant: 5),
viewTitleLabel.centerYAnchor.constraint(equalTo: self.centerYAnchor),
viewTitleLabel.heightAnchor.constraint(equalToConstant: 30),
viewTitleLabel.widthAnchor.constraint(equalToConstant: 90),
countryCollectionView.leadingAnchor.constraint(equalTo: viewTitleLabel.trailingAnchor, constant: 10),
countryCollectionView.centerYAnchor.constraint(equalTo: self.centerYAnchor),
countryCollectionView.trailingAnchor.constraint(equalTo: self.trailingAnchor, constant: -10),
countryCollectionView.heightAnchor.constraint(equalToConstant: 50)
])
Constraints on container view when adding to UIViewContoller:
countryCountView.translatesAutoresizingMaskIntoConstraints = false
countryCountView.isUserInteractionEnabled = true
view.addSubview(countryCountView)
NSLayoutConstraint.activate([
countryCountView.leadingAnchor.constraint(equalTo: view.leadingAnchor, constant: 0),
countryCountView.trailingAnchor.constraint(equalTo: view.trailingAnchor),
countryCountView.topAnchor.constraint(equalTo: headerContainer.bottomAnchor),
countryCountView.heightAnchor.constraint(equalToConstant: 60)
])
My UICollectionViewCell:
override init(frame: CGRect) {
countryNameLabel = UILabel()
countryUserCountLabel = UILabel()
super.init(frame: frame)
contentView.addSubview(countryNameLabel)
countryNameLabel.translatesAutoresizingMaskIntoConstraints = false
countryNameLabel.font = UIFont.boldSystemFont(ofSize: 13)
countryNameLabel.textColor = .white
contentView.addSubview(countryUserCountLabel)
countryUserCountLabel.translatesAutoresizingMaskIntoConstraints = false
countryUserCountLabel.font = UIFont.systemFont(ofSize: 13)
countryUserCountLabel.textColor = .white
NSLayoutConstraint.activate([
countryNameLabel.leadingAnchor.constraint(equalTo: self.leadingAnchor, constant: 5),
countryNameLabel.heightAnchor.constraint(equalToConstant: 30),
countryNameLabel.topAnchor.constraint(equalTo: self.topAnchor, constant: 5),
countryNameLabel.bottomAnchor.constraint(equalTo: self.bottomAnchor, constant: -5),
// countryNameLabel.widthAnchor.constraint(greaterThanOrEqualToConstant: 0),
// countryNameLabel.widthAnchor.constraint(equalToConstant: 20),
countryUserCountLabel.leadingAnchor.constraint(equalTo: countryNameLabel.trailingAnchor, constant: 5),
countryUserCountLabel.topAnchor.constraint(equalTo: self.topAnchor, constant: 5),
countryUserCountLabel.bottomAnchor.constraint(equalTo: self.bottomAnchor, constant: -5),
countryUserCountLabel.trailingAnchor.constraint(equalTo: self.trailingAnchor, constant: -5),
countryUserCountLabel.heightAnchor.constraint(equalToConstant: 30),
// countryUserCountLabel.widthAnchor.constraint(equalToConstant: 40)
])
}
Height returned by delegate method:
func collectionView(_ collectionView: UICollectionView, layout collectionViewLayout: UICollectionViewLayout, sizeForItemAt indexPath: IndexPath) -> CGSize {
return CGSize(width: 70, height: 40)
// return CGSize.zero
}
Before scrolling:
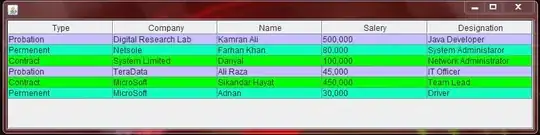
After scrolling:
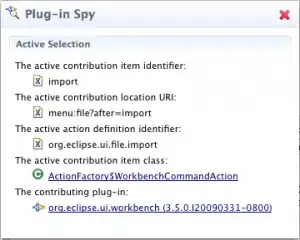
Edit:
Setting collectionView.showsHorizontalScrollIndicator = false
does not help.
Also setting layout.estimatedItemSize = CGSize.zero
fixes the shifting up but stops the cells from resizing correctly.
