Edit at bottom to include given data.
If there's a way to get the graph's axis transformed like maybe is more intuitive I don't know. I couldn't figure that out.
As other's mentioned having some sample data in your code would be helpful so that solutions could use it instead of having to guess and/or make up our own. Including a picture of what you have and/or want is also good.
Something like:
R> head(SLEEP2)
...
Anyways, here's one way of doing it. It's not at all elegant but it works.
First, make some "untransformed" data that peaks at midnight and plot it. I'm guessing this looks something like what you have already.
library(tidyverse)
library(lubridate)
start <- ymd_hm("20210101-0000")
# Make some pretend data. It's value is zero during the "day"
# and peaks around midnight.
secsday <- 60*60*12
df <- tibble(date_time = seq(start, start %m+% weeks(2), by=200)) %>%
mutate(time = hms::as_hms(date_time),
date = factor(date(date_time)),
values = jitter(cos(day(date_time)/30+pi*as.numeric(time)/secsday), amount=1/40),
values = ifelse(values>0, values, 0)
)
# Plot original data.
ggplot(df, aes(time, values, colour=date)) +
geom_line() +
scale_x_time(breaks=hours(seq(0,24,6)), labels=c("midnight","6AM","noon","6pm","midnight")) +
ggtitle('pre-transform')
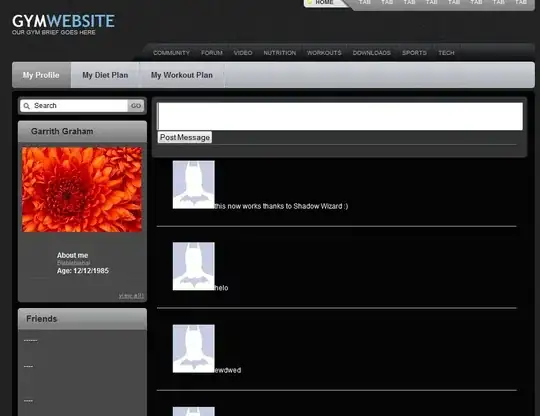
Next, translate AM to PM and PM to AM.
# Transform times so AM is PM and PM is AM (swap around noon).
dt <- df %>% mutate(time=
ifelse(time>hm("12:00"), hms::as_hms(time)-secsday, hms::as_hms(time)+secsday)
)
ggplot(dt, aes(time, values, colour=date)) +
geom_line() +
scale_x_time(breaks=hours(seq(0,24,6)), labels=c("noon", "6PM", "midnight", "6AM", "noon")) +
ggtitle('post-transform')
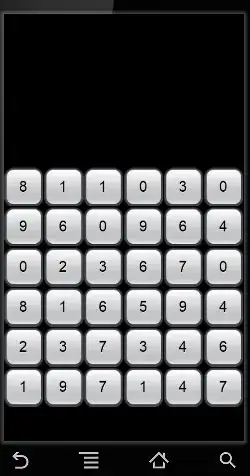
I'd like to know if there's a way of doing this by only adjusting the axis instead of the data.
With the data you provided and using bars:
library(lubridate)
dat <- data.frame(Jour=1:5,
date=seq.Date(as.Date("2020-10-01"), as.Date("2020-10-05"), "day"),
sleeptime=c("22:30","21:10","23:00","23:00", "23:20"),
waketime= c("6:30", "7:00", "7:30", "6:25","7:10"))
# make fake dates for sleep and wake
dat <- dat %>% mutate(
sleep = ymd_hm(paste(dat[1,]$date, sleeptime)),
wake = ymd_hm(paste(dat[1,]$date, waketime)),
wake = wake + ddays(ifelse(lubridate::hm(waketime)<lubridate::hm(sleeptime), 1, 0))
)
ggplot(dat, aes(xmin=sleep, xmax=wake)) +
scale_x_datetime(breaks=ymd(dat[1,]$date) + hours(seq(12,36,length.out=5)),
labels=c("noon", "6PM", "midnight", "6AM", "noon"),
limits=ymd(dat[1,]$date) + hours(seq(12,36,length.out=2))
) +
geom_rect(ymin=0.25, ymax=0.75, fill='darkgreen') +
ylim(0,1) +
facet_grid(rows = vars(date)) +
theme(axis.title = element_blank()) +
theme(axis.text.y = element_blank(),
axis.ticks = element_blank()
)
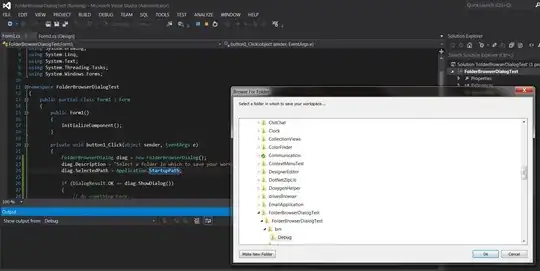