I added a slightly smaller PictureBox that I want to be the "real" background so it will create a neat border between the main form and the background image.
That background PictureBox
is causing this. The smaller picture boxes with the transparent back color need to be placed directly into a container with a background image and nothing in between. For example, you could remove the background picture box and override the Form's OnPaint
method to draw the background image and leave some margins:
using System;
using System.Drawing;
using System.Windows.Forms;
public partial class LauncherForm : Form
{
public LauncherForm() : base()
{
DoubleBuffered = true;
ResizeRedraw = true;
BackColor = Color.Black;
Padding = new Padding(20);
ControlBox = false;
FormBorderStyle = FormBorderStyle.None;
}
private Image bgImage;
public new Image BackgroundImage
{
get => bgImage;
set { bgImage = value; Invalidate(); }
}
protected override void OnPaint(PaintEventArgs e)
{
var g = e.Graphics;
var r = new Rectangle(Padding.Left, Padding.Top,
Width - Padding.Left - Padding.Right,
Height - Padding.Top - Padding.Bottom);
g.Clear(BackColor);
if (BackgroundImage != null)
g.DrawImage(BackgroundImage, r);
g.DrawRectangle(Pens.DimGray, r);
}
}
Another option is to do the same but with a Panel
control to host the other picture boxes:
- Select your project.
- Right click and select
Add -> Class..
.
- In the add dialog, rename the class to
LauncherPanel.cs
and hit the Add
button.
- Replace the class block only (keep the namespace) in the new class with the code below.
- Save and rebuild.
- Open the designer, check the top of the
ToolBox
window, you'll find the new control LauncherPanel
under the components of your project.
- Drop an instance into the designer.
- Select the control and press
F4
key to activate the Properties
window. Set the Image
property and optionally the BorderColor
and BorderWidth
properties.
- Add the other picture boxes into the
launcherPanel1
or whatever you name it.
using System;
using System.Drawing;
using System.Windows.Forms;
using System.ComponentModel;
// Within the namespace of your project..
[DesignerCategory("")]
public class LauncherPanel : Panel
{
public LauncherPanel() : base()
{
DoubleBuffered = true;
ResizeRedraw = true;
BackColor = Color.Black;
}
private Image bgImage;
public Image Image
{
get => bgImage;
set { bgImage = value; Invalidate(); }
}
private Color borderColor = Color.DimGray;
[DefaultValue(typeof(Color), "DimGray")]
public Color BorderColor
{
get => borderColor;
set { borderColor = value; Invalidate(); }
}
private int borderWidth = 1;
[DefaultValue(1)]
public int BorderWidth
{
get => borderWidth;
set { borderWidth = value; Invalidate(); }
}
protected override void OnPaint(PaintEventArgs e)
{
var g = e.Graphics;
var r = ClientRectangle;
r.Width--;
r.Height--;
g.Clear(BackColor);
if (Image != null)
g.DrawImage(Image, r);
using (var pn = new Pen(borderColor, borderWidth))
g.DrawRectangle(pn, r);
}
}
Choosing the Panel
option, you will get something like this at design time:
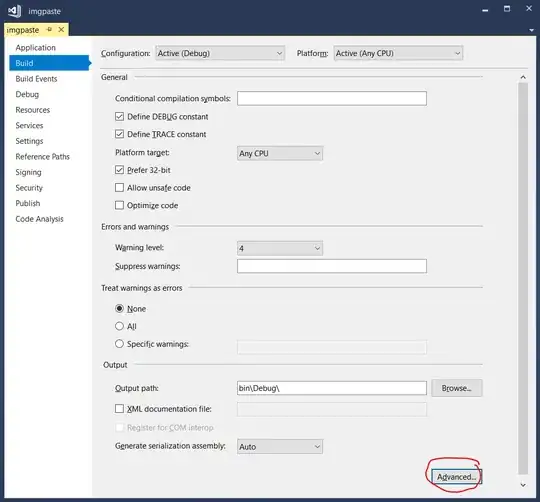
And like this at runtime:
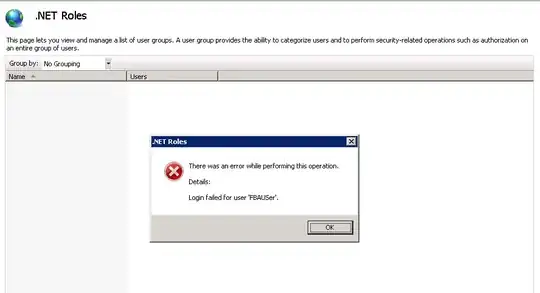
Make sure you have transparent PNG
images for the picture boxes in the Panel
.