I decided to write a medium.com article to answer this question and did just that. Here is the solution looks like and here is the code too. Here is the article and well the solution.
https://marklucking.medium.com/a-real-world-alignment-challenges-in-swiftui-2-0-ff440dceae5a
In short I setup the four labels with the correct alignment and then aligned the four containers with each other.
In this code I included a slider so that you can better understand how it works.
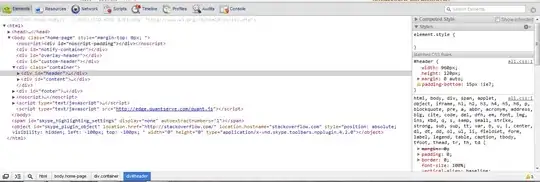
import SwiftUI
struct ContentView: View {
private var newAlignment1H: HorizontalAlignment = .leading
private var newAlignment1V: VerticalAlignment = .top
private var newAlignment2H: HorizontalAlignment = .trailing
private var newAlignment2V: VerticalAlignment = .top
@State private var zeroX: CGFloat = 160
var body: some View {
VStack {
ZStack(alignment: .theAlignment) {
HStack {
Label {
Text("Team 1")
.font(.system(size: 16, weight: .semibold, design: .rounded))
} icon: {
Image(systemName:"seal")
.resizable()
.scaledToFit()
.frame(width: 30)
}.labelStyle(HorizontalLabelStyle())
}
.border(Color.blue)
.alignmentGuide(.theHorizontalAlignment, computeValue: {d in zeroX})
// .alignmentGuide(.theHorizontalAlignment, computeValue: {d in d[self.newAlignment2H]})
// .alignmentGuide(.theVerticalAlignment, computeValue: {d in d[self.newAlignment1V]})
HStack {
Text("65")
.font(.system(size: 16, weight: .semibold, design: .rounded))
.background(Color.red.opacity(0.2))
.frame(width: 128, height: 32, alignment: .trailing)
Text("Final")
.font(.system(size: 16, weight: .semibold, design: .rounded))
.background(Color.red.opacity(0.2))
.frame(width: 48, height: 32, alignment: .leading)
}
.border(Color.green)
}
ZStack(alignment: .theAlignment) {
HStack {
Label {
Text("Team No 2")
.font(.system(size: 16, weight: .semibold, design: .rounded))
} icon: {
Image(systemName:"seal")
.resizable()
.scaledToFit()
.frame(width: 30)
}.labelStyle(HorizontalLabelStyle())
}
// .alignmentGuide(.theHorizontalAlignment, computeValue: {d in d[self.newAlignment2H]})
// .alignmentGuide(.theVerticalAlignment, computeValue: {d in d[self.newAlignment1V]})
.alignmentGuide(.theHorizontalAlignment, computeValue: {d in zeroX})
.border(Color.pink)
HStack {
Text("70")
.font(.system(size: 16, weight: .semibold, design: .rounded))
.background(Color.red.opacity(0.2))
.frame(width: 128, height: 32, alignment: .trailing)
Text("")
.background(Color.red.opacity(0.2))
.frame(width: 48, height: 32, alignment: .leading)
}
.border(Color.orange)
}
VStack {
Slider(value: $zeroX, in: 0...200, step: 10)
Text("\(zeroX)")
}
}
}
}
struct VerticalLabelStyle: LabelStyle {
func makeBody(configuration: Configuration) -> some View {
VStack(alignment: .center, spacing: 8) {
configuration.icon
configuration.title
}
}
}
struct HorizontalLabelStyle: LabelStyle {
func makeBody(configuration: Configuration) -> some View {
HStack(alignment: .center, spacing: 8) {
configuration.icon
configuration.title
}
}
}
extension VerticalAlignment {
private enum TheVerticalAlignment : AlignmentID {
static func defaultValue(in d: ViewDimensions) -> CGFloat {
return d[VerticalAlignment.top]
}
}
static let theVerticalAlignment = VerticalAlignment(TheVerticalAlignment.self)
}
extension HorizontalAlignment {
private enum TheHorizontalAlignment : AlignmentID {
static func defaultValue(in d: ViewDimensions) -> CGFloat {
return d[HorizontalAlignment.leading]
}
}
static let theHorizontalAlignment = HorizontalAlignment(TheHorizontalAlignment.self)
}
extension Alignment {
static let theAlignment = Alignment(horizontal: .theHorizontalAlignment, vertical: .theVerticalAlignment)
}
struct ContentView_Previews: PreviewProvider {
static var previews: some View {
ContentView()
}
}