tl;dr
LocalDateTime
.parse(
"2014/10/29 18:10:45".replace( " " , "T" )
)
.atOffset( ZoneOffset.UTC )
.toInstant()
.toEpochMilli()
Need epoch reference
Representing a moment as a count of milliseconds requires a point in time as an epoch reference. You need to state the reference needed in your situation. I will assume the commonly used point of first moment of 1970 in UTC. But there are a couple dozen other points used by various systems. So you need to find out the meaning of your own data.
Need time zone or offset
Determining a moment requires more than a date and a time-of-day. You also need the context of a time zone or offset-from-UTC. Again, you need to specify this but did not. Is your example of ten minutes past six in the evening in Tokyo Japan, Toulouse France, or Toledo Ohio US? I will assume you mean an offset of zero hours-minutes-seconds. But again, you need to find out the meaning of your own data.
Avoid legacy date-time classes
Never use SimpleDateFormat
, Date
, or the other terrible date-time classes that were supplanted years ago by the modern java.time classes defined in JSR 310.
Date versus moment
Your Question in confused, referring to a date-only value as well as a date with time-of-day represented as milliseconds. These are two different kinds of data.
If representing a date-only, use LocalDate
in Java and a type in your database akin to the SQL-standard DATE
. I will ignore this date-only, and focus on tracking a moment.
Example code
Parse your input as LocalDateTime
, after complying with standard ISO 8601 format by replacing SPACE in middle with a T
.
A LocalDateTime
does not represent a moment, is not a point on the timeline. You need to discover the zone/offset intended for you input, and apply. Apply the time zone intended for your input, to produce a ZonedDateTime
. Or, if UTC (an offset of zero) was intended, apply a ZoneOffset
to get an OffsetDateTime
object. At this point we have determined a moment.
Extract a Instant
object from the OffsetDateTime
. Interrogate for a count of milliseconds since the epoch reference of 1970-01-01T00:00Z
.
String myDate = "2014/10/29 18:10:45".replace( " " , "T" ) ; // Comply with ISO 8601 standard formatting.
LocalDateTime ldt = LocalDateTime.parse( input ) ;
OffsetDateTime odt = ldt.atOffset( ZoneOffset.UTC ) ; // Assuming your data was intended to represent a moment as seen in UTC, with an offset of zero hours-minutes-seconds.
Instant instant = odt.toInstant() ; // Basic building-block class in java.time, representing a moment as seen in UTC.
long millisecondsSinceEpoch1970 = instant.toEpochMilli() ;
Your title mentions Firebase, but that seems irrelevant to your Question. so I will ignore that topic.
All the content in this Answer has been covered many times already on Stack Overflow. Search to learn more.
About java.time
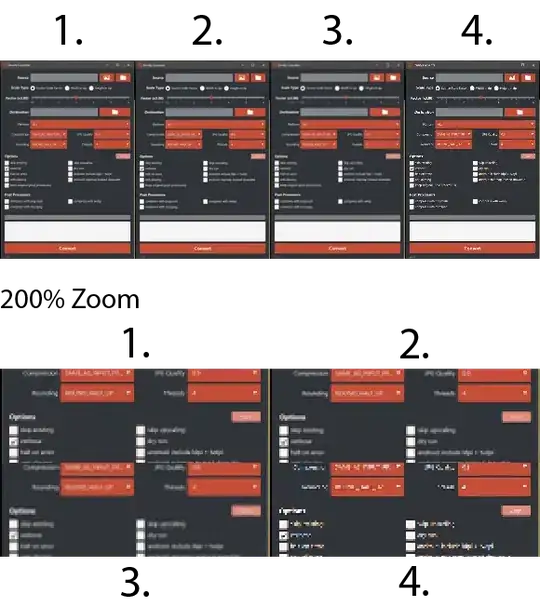
The java.time framework is built into Java 8 and later. These classes supplant the troublesome old legacy date-time classes such as java.util.Date
, Calendar
, & SimpleDateFormat
.
To learn more, see the Oracle Tutorial. And search Stack Overflow for many examples and explanations. Specification is JSR 310.
The Joda-Time project, now in maintenance mode, advises migration to the java.time classes.
You may exchange java.time objects directly with your database. Use a JDBC driver compliant with JDBC 4.2 or later. No need for strings, no need for java.sql.*
classes. Hibernate 5 & JPA 2.2 support java.time.
Where to obtain the java.time classes?