Given your sample and approach, the answer you were probably initially looking for is provided by @sai kiran.
That is, you're doing ToString()
of Form.Location
(which is a System.Drawing.Point
type) and you're writing that to a file in your approach. Note: the output string of ToString()
is (X=?,Y=?)
, where ?
is numerical characters representing the value.
Given that you are storing Location
Point
value that way (and not the serialized form of Point
) and In order to achieve your desire result, you'll need to read that file and parse\handle the string represented value, constructing a new Point
type instance from it, then assign it to Location
before the form is shown.
There is NO standard Point.Parse
method for this ToString
string representation of Point
(that I'm aware of), so you'll have to handle it with string manipulations and extrapolations. Note: if it was represented as "0, 0"
, you could deserialize it to Point
type instance
Having said that, you can accomplish this task without specifically implementing File\Stream reads\writes nor parsing the extrapolated values of a string by using Application Settings and control Property Binding.
And actually, Using Application Settings and Property Binding is my recommended approach and answer here. I'll provide some instruction on doing that:
You'll define a Setting (for example "Form1LocationPointUserSetting"
) as a System.Drawing.Point
in the scope of User. Since Point
is a value type, it will have a default value - (0, 0)
You can define setting in a couple ways, but the easiest is probably using the Settings Designer tool of your IDE (it will generate code and update App.config)
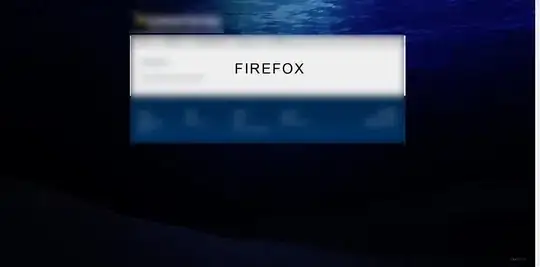
After saving settings designer changes (with CTRL+S to save all), you will be able to both programmatically reference to the Setting property name in code and use it in control property bindings (as it relates to the Type of Property it is). Note: not all control properties support binding in this manner, and some control properties that support bind may not implement\update with INotifyProperty
changes.
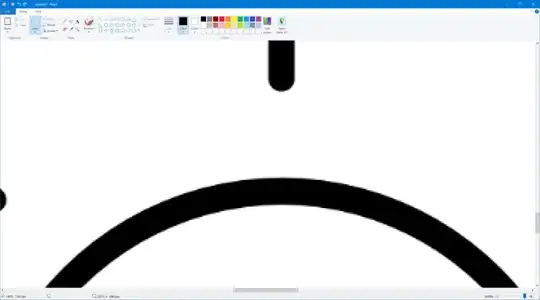
Lastly, you'll want to persist any changes you've made to settings. So you'll call Property.Settings.Default.Save()
during some event. (such as form closing event.)
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
FormClosing += Form1_FormClosing;
}
private void Form1_FormClosing(object sender, FormClosingEventArgs e)
{
//Save Settings
Properties.Settings.Default.Save();
}
}