If you're performing any sort of color-based segmentation of images, you may find it easier to convert to HSV color space first to select specific color ranges. I outline how you can do this sort of thing in an answer I gave to a similar question. The steps you would likely want to follow would be the following:
- Convert to HSV and create a binary mask by selecting pixels with hues within a green color range and with a minimum amount of saturation and value.
- Erode the resulting mask by a certain amount to remove small spurious clusters.
- Dilate the eroded mask to get back to a smoother edge for your selected pixels.
I can't give an exact example of how you would apply this analysis to your data since the image you provide in the question actually has a higher resolution than the data it displays, but here's a general solution that uses the functions RGB2HSV, IMERODE, and IMDILATE (the last two are from the Image Processing Toolbox):
rgbImage = imread('data.jpg'); %# Load the RGB image
hsvImage = rgb2hsv(rgbImage); %# Convert to HSV color space
hPlane = 360.*hsvImage(:,:,1); %# Get the hue plane, scaled from 0 to 360
vPlane = hsvImage(:,:,3); %# Get the value plane
mask = (hPlane >= 80) & (hPlane <= 140) & (vPlane >= 0.3); %# Select a mask
SE = strel('disk',10); %# Create a disk-shaped element
mask = imdilate(imerode(mask,SE),SE); %# Erode and dilate the mask
And here's some code to visualize the edges created by the above analysis:
edgeMask = mask-imerode(mask,strel('disk',1)); %# Create an edge mask
edgeImage = zeros([size(edges) 3]); %# Create an RGB image for the edge
edgeImage(find(edgeMask)) = 1; %# that's colored red
image(rgbImage); %# Plot the original image
hold on;
image(edgeImage,'AlphaData',edgeMask); %# Plot the edge image over it
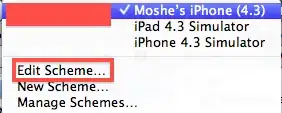