I've done this before but I'm using electron-webpack
in addition to what you already have:
electron ^11.2.0
electron-builder ^22.9.1
electron-webpack ^2.8.2
webpack ^4
The idea is to expose a variable via webpack.DefinePlugin
and you can do this for both your main and renderer processes.
With electron-webpack
you can provide a webpack
configuration for both processes:
// webpack.main.config.js
const webpack = require('webpack');
module.exports = {
plugins: [ new webpack.DefinePlugin({__BURRITO__: true}) ]
};
// webpack.renderer.config.js
const webpack = require('webpack');
module.exports = {
plugins: [ new webpack.DefinePlugin({__TACO__: true}) ]
};
These two files are exposed to electron-webpack
via a bit of configuration in package.json
:
{
…
"electronWebpack": {
"main": {
"webpackConfig": "webpack.main.config.js"
},
"renderer": {
"webpackConfig": "webpack.renderer.config.js"
}
}
}
Then in your main process:
// src/main/index.js
const {app, BrowserWindow} = require('electron')
app.whenReady().then(() => {
const mainWindow = new BrowserWindow({webPreferences: {nodeIntegration: true}});
console.log(__BURRITO__ ? "" : "");
mainWindow.loadURL(`http://localhost:${process.env.ELECTRON_WEBPACK_WDS_PORT}`);
});
Two things:
The .loadURL
bit looks funny but that's only because I'm using electron-webpack
default html template for the renderer. During development it is served through this local server.
You shouldn't need to enable the nodeIntegration
flag. That is only because electron-webpack
default renderer template uses a few require
statements here and there.
If your renderer process includes some scripts they'll have access to your at-compile-time variable too. Here again I'm leveraging electron-webpack
default template which requires src/renderer/index.js
automatically:
// src/renderer/index.js
if (__TACO__) {
document.body.innerHTML = ""
}
When you run this via electron-webpack dev
then you can see two things:
- A burrito is seen in the output of the main process
- A taco is seen in the html of the renderer process
This shows that electron-webpack
successfully injected two variables at compile time.
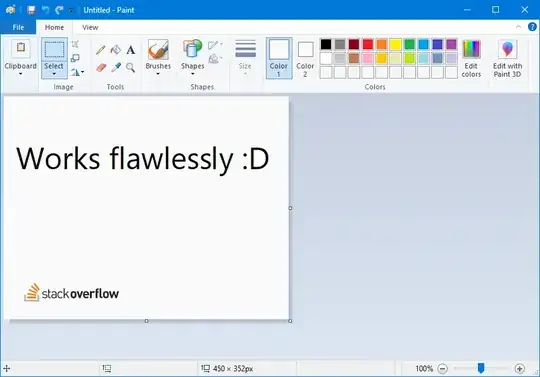