Two Issues will fix your problem. Add tabindex="0"
to your DIV
then handle the events below
<!-- language: html -->
// 1
<div (blur)="onBlur()"> // tabindex="0" gets inserted by HostBinding
// recommend you use an input vs. DIV when user is entering data
the second issue is because there are two events happening
as a result of your navigation you need to handle both. For firefox look here for the eventname. Once you import HostListener
& HostBinding
<!-- language: typescript -->
// 2
import { Component, HostListener, HostBinding } from '@angular/core';
- Handle the
focus event
both focus in and blur
for exit
- Handle the
blur event
as you want
<!-- language: typescript -->
// 3 -- you should be good :)
export class HotComponent {
focusOutFunction() {
console.log('focusOutFunction called');
.. your logic here
}
focusFunction() {
console.log('focusFunction called');
..
}
blurFunction()
{
console.log('blur called');
..
}
// you dont need to manually add it to HTML if you do this.
@HostBinding('attr.tabindex') tabindex = '0';
}
Another option 2: if you have want to handle focusOut
<!-- language: html -->
<input tabindex="0" type="text" (focusout)="onFocusOutEvent($event)" />
// in your component explicitly handle the event
onFocusOutEvent(event: any){
console.log(event.target.value);
}
A simple working sample with the events working for me, Please see console log below pic... it works. Can you please disable all your plugins and try.
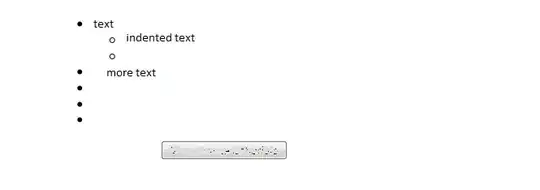