I think the primary issue is that your groupby
doesn't reflect the grouping shown in the desired plot. Including the 'year'
column means you keep separate dates from different years. But the plot looks like it is trying to show what an average year looks like; i.e. dates from different years should be binned together.
So I would instead try just grouping by month and day:
dailyMean = df.groupby(['month','day'])['births'].mean().reset_index()
# month day births
# 0 1 1.0 4009.225
# 1 1 2.0 4247.400
# 2 1 3.0 4500.900
# 3 1 4.0 4571.350
# 4 1 5.0 4603.625
And then (I would agree with others that) converting to timestamps might be the most flexible for plotting. You could make timestamps out of your data using an arbitrary year (I choose 2000
as it is a leap year):
dates = pd.to_datetime(dict(year=2000, month=dailyMean['month'], day=dailyMean['day']))
Then you can just plot the births against the dates, and use matplotlib.dates
to do a little additional formatting:
import matplotlib.dates as mdates
import matplotlib.pyplot as plt
import pandas as pd
plt.style.use('seaborn')
fig, ax = plt.subplots()
ax.plot(dates, dailyMean['births'])
ax.xaxis.set_major_formatter(mdates.DateFormatter('%b'))
ax.xaxis.set_major_locator(mdates.MonthLocator())
ax.set_xlim(dates.iloc[0], dates.iloc[-1])
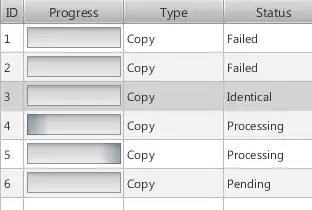
Note that this looks a little different from your expected graph; I think the major thing is that mine includes February 29th and it looks like yours doesn't. It looked like your data came from here. I had to do some cleaning, and maybe you have done different. But here's my full example:
import matplotlib.dates as mdates
import matplotlib.pyplot as plt
import pandas as pd
# load the data from https://www.kaggle.com/asauve/cdc-us-births-data-19692008
df = pd.read_csv('births.csv')
# get what each day of the year looks like on average
dailyMean = df.groupby(['month','day'])['births'].mean().reset_index()
# clean data by removing rows that aren't real dates
dr = pd.date_range('01-01-2020', '12-31-2020', freq='D')
realdates = pd.Series(tuple(zip(dr.month, dr.day)))
dfdates = pd.Series(tuple(zip(dailyMean['month'], dailyMean['day'].astype(int))))
dailyMean = dailyMean[dfdates.isin(realdates)]
# make a new dates series for plotting
dates = pd.to_datetime(dict(year=2000, month=dailyMean['month'], day=dailyMean['day']))
# plot
plt.style.use('seaborn')
fig, ax = plt.subplots()
ax.plot(dates, dailyMean['births'])
ax.xaxis.set_major_formatter(mdates.DateFormatter('%b'))
ax.xaxis.set_major_locator(mdates.MonthLocator())
ax.set_xlim(dates.iloc[0], dates.iloc[-1])