What you want to do is surprisingly difficult, which is why most people would not choose to do it that way :)
The closest solution I could find uses ggplot2
and a lot of other packages. It's possible to do the following:
- Create a summary table of the regression model using
stargazer::stargazer()
- Convert that to a PNG image file using
kableExtra::as_image()
- Convert the PNG to a grob using
grid::rasterGrob()
- Use
ggplot2::annotation_custom()
to embed the table-as-image-as-grob into a ggplot2
chart
Note that as_image()
requires some other packages and an installation of phantomjs.
Here's an example:
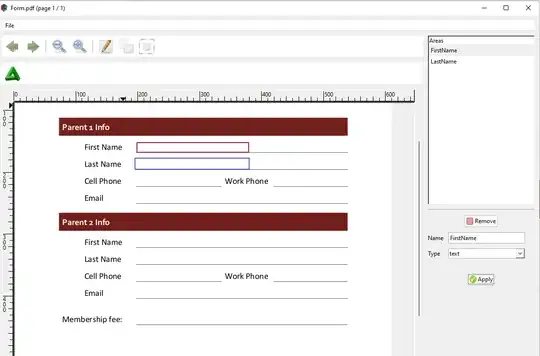
However, there are other solutions that might be better such as a simple summary using ggpubr::stat_regline_equation()
or adding a table grob using the output of broom::tidy()
.
I think the simplest way to demonstrate all the options is in a RMarkdown file. Here is the code to copy into an RMarkdown file which you can knit in RStudio.
---
title: "Regression"
author: "Neil Saunders"
date: "27/01/2021"
output:
html_document:
toc: yes
---
```{r setup, include=FALSE}
knitr::opts_chunk$set(echo = FALSE,
message = FALSE,
fig.path = "figures/")
library(ggplot2)
library(ggpubr)
library(broom)
library(gridExtra)
library(kableExtra)
library(grid)
library(sjPlot)
library(stargazer)
library(png)
theme_set(theme_bw())
```
# The model
```{r echo=TRUE}
linearMod <- cars %>%
lm(dist ~ speed, data = .)
```
# Visualizations
## Add equation and adjusted R-squared to a plot
```{r}
cars %>%
ggplot(aes(speed, dist)) +
geom_point() +
geom_smooth(method = "lm") +
stat_regline_equation(
aes(label = paste(..eq.label.., ..adj.rr.label.., sep = "~~~~"))
)
```
## Add tidy summary table to a plot
```{r}
linearMod_tidy <- tidy(linearMod)
cars %>%
ggplot(aes(speed, dist)) +
geom_point() +
geom_smooth(method = "lm") +
annotation_custom(tableGrob(linearMod_tidy,
theme = ttheme_default(base_size = 10)),
xmin = 0, ymin = 90)
```
## Add tabular summary and plot side-by-side
### stargazer
:::::: {.columns}
::: {.column width="48%" data-latex="{0.48\textwidth}"}
```{r}
cars %>%
ggplot(aes(speed, dist)) +
geom_point() +
geom_smooth(method = "lm")
```
:::
::: {.column width="4%" data-latex="{0.04\textwidth}"}
\
<!-- an empty Div (with a white space), serving as
a column separator -->
:::
:::::: {.column width="48%" data-latex="{0.48\textwidth}"}
```{r results='asis'}
stargazer(linearMod, type = "html")
```
:::
::::::
### tab\_model
:::::: {.columns}
::: {.column width="48%" data-latex="{0.48\textwidth}"}
```{r echo=FALSE,}
cars %>%
ggplot(aes(speed, dist)) +
geom_point() +
geom_smooth(method = "lm")
```
:::
::: {.column width="4%" data-latex="{0.04\textwidth}"}
\
<!-- an empty Div (with a white space), serving as
a column separator -->
:::
:::::: {.column width="48%" data-latex="{0.48\textwidth}"}
```{r}
tab_model(linearMod)
```
:::
::::::
## Add stargazer table to a plot
```{r}
imgfile <- stargazer(linearMod, type = "html") %>%
as_image()
img <- readPNG(imgfile)
g <- rasterGrob(img, interpolate = TRUE, width = 0.5, height = 0.5)
cars %>%
ggplot(aes(speed, dist)) +
geom_point() +
geom_smooth(method = "lm") +
annotation_custom(g, xmin = 1, xmax = 15, ymin = 50, ymax = 130)
```