You are trying to sort the rows in place, and spreadsheet formulas can't do that as it will cause circular dependency.
I recommend using Apps Script instead:
function myFunction() {
var sheet = SpreadsheetApp.getActiveSheet();
var lastColumn = sheet.getLastColumn();
// Sort 1st row (B1 - E1)
var firstValues = sheet.getRange(1, 2, 1, lastColumn - 1).getValues().flat();
sheet.getRange(1, 2, 1, lastColumn - 1).setValues([sortWithIndices(firstValues)]);
// Using loop, sort 2nd - 10th row based on the
// movement of indices when sorting the 1st row
var i = 2;
var lastRow = 10;
while(i <= lastRow) {
var values = sheet.getRange(i, 2, 1, lastColumn - 1).getValues().flat();
var tempValues = sheet.getRange(i, 2, 1, lastColumn - 1).getValues().flat();
for (var index = 0; index < values.length; index++) {
values[index] = tempValues[firstValues.sortIndices[index]];
}
sheet.getRange(i, 2, 1, lastColumn - 1).setValues([values]);
i++;
}
}
function sortWithIndices(toSort) {
for (var i = 0; i < toSort.length; i++) {
toSort[i] = [toSort[i], i];
}
toSort.sort(function(left, right) {
// < means ascending
// > means descending
return left[0] < right[0] ? -1 : 1;
});
toSort.sortIndices = [];
for (var j = 0; j < toSort.length; j++) {
toSort.sortIndices.push(toSort[j][1]);
toSort[j] = toSort[j][0];
}
return toSort;
}
It got very different from simply sorting per row since we are now sorting other rows based on the first row.
So basically, I created a function that is saving the changes in indices after sorting the first row (firstValues.sortIndices
). This is an array that contains the index changes when the first row was sorted.
After getting that, it becomes easier as we only need to swap the values of the succeeding rows (2-10) based on the firstValues.sortIndices
.
It happens inside the for
loop.
Sample Output:
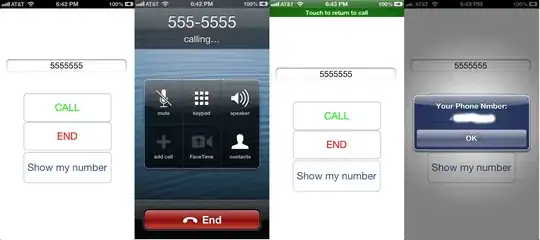
This is one of the possible answers I can think off, and is actually the simplest to do but lengthy to apply.
One possible answer to this is swapping the whole columns instead and I do think that would be better and faster than this.
Reference: