There's a pretty simple feature for it. It took me also, an hour to figure out it.
The chartConfig has a field called, useShadowColorFromDataSet -> Boolean
Here's the documentation -
https://github.com/indiespirit/react-native-chart-kit
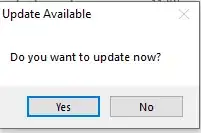
Just declare that in the chartConfig and then use the Color field in datasets and you'll be done.
Here's a sample code snippet ->
import * as React from 'react';
import { Text, View, StyleSheet } from 'react-native';
import Constants from 'expo-constants';
import {
LineChart,
BarChart,
PieChart,
ProgressChart,
ContributionGraph,
StackedBarChart,
} from 'react-native-chart-kit';
import { Dimensions } from 'react-native';
const screenWidth = Dimensions.get('window').width;
// You can import from local files
import AssetExample from './components/AssetExample';
// or any pure javascript modules available in npm
import { Card } from 'react-native-paper';
export default function App() {
return (
<View>
<Text>Bezier Line Chart</Text>
<LineChart
data={{
labels: ['January', 'February', 'March', 'April', 'May', 'June'],
datasets: [
{
data: [9, 7, 6, 4, 2, 5],
strokeWidth: 2,
color: (opacity = 1) => purple,
// optional
},
{
data: [9, 4, 6, 8, 8, 2],
strokeWidth: 2,
color: (opacity = 1) => black,
colors: 'purple' // optional
},
{
data: [9, 4, 7, 8, 2, 4],
strokeWidth: 3,
color: (opacity = 1) => blue, // optional
},
],
}}
width={Dimensions.get('window').width} // from react-native
height={220}
yAxisLabel="$"
yAxisSuffix="k"
yAxisInterval={1} // optional, defaults to 1
chartConfig={{
backgroundColor: 'grey',
backgroundGradientFrom: 'grey',
backgroundGradientTo: 'grey',
decimalPlaces: 2, // optional, defaults to 2dp
color: (opacity = 1) => rgba(255, 255, 255, ${opacity}),
labelColor: (opacity = 1) => rgba(255, 255, 255, ${opacity}),
style: {
borderRadius: 16,
},
propsForDots: {
r: '',
},
propsForBackgroundLines: {
color: 'black',
stroke: 'black',
strokeDasharray:[],
},
useShadowColorFromDataset: true
}}
bezier
style={{
marginVertical: 8,
borderRadius: 16,
}}
/>
</View>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
paddingTop: Constants.statusBarHeight,
backgroundColor: '#ECF0F1',
padding: 8,
},
paragraph: {
margin: 24,
fontSize: 18,
fontWeight: 'bold',
textAlign: 'center',
},
});
Well, it was always present in the docs. Which teaches, that always read the docs properly. It took me an hour to figure it out.