You should override paintComponent(Graphics g)
and draw the image therein. In this case, you should do this for the JPanel
component (I think? If not, do this for the JComponent
(s) you're referring to). Also, since Swing is not thread-safe, ensure these modifications are performed in the EDT
.
EXAMPLE
public class Demo{
private static BufferedImage bi;
public static void main(String[] args){
try{
loadImage();
SwingUtilities.invokeLater(new Runnable(){
@Override
public void run(){
createAndShowGUI();
}
});
}
catch (IOException e){
// handle exception
}
}
private static void loadImage() throws IOException{
bi = ImageIO.read(new File("src/resource/braveheart.PNG"));
}
private static void createAndShowGUI(){
final JFrame frame = new JFrame();
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
final JPanel panel = new JPanel(){
@Override
protected void paintComponent(Graphics g){
Graphics g2 = g.create();
g2.drawImage(bi, 0, 0, getWidth(), getHeight(), null);
g2.dispose();
}
@Override
public Dimension getPreferredSize(){
return new Dimension(bi.getWidth(), bi.getHeight());
}
};
frame.add(panel);
frame.pack();
frame.setLocationRelativeTo(null);
frame.setVisible(true);
}
}
OUTPUT
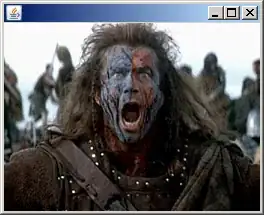
It's important to keep in mind that this example ignores rendering hints, so when you maximize the JFrame
, the image quality will be very poor. :)
EDIT
When answering this question, I assumed you had a basic understanding of Swing. I suppose I assumed too much. It is important to mention that all components should be added to the top-level container before it's been realized (i.e. made visible). This will ensure that everything is rendered without having to resize your frame. As others have suggested, you could have simply used a JLabel
to render the image, and then added it to your JPanel
. Instead, I promoted custom painting, which is perfectly acceptable, and to me, cleaner.