If the question is what will happen if the malloc
fails and bar
will be assigned NULL
, then the answer is: nothing will happen when free
is called. free
function checks if the pointer passed is NULL
. If the pointer is NULL
no action is taken. So there is no UB here.
As a general remark: it is safer (or at least less error-prone) if instead of types the actual objects are used:
struct foo * bar = malloc(sizeof(*bar));
#EDIT#
OPs comment clarifies the question. The size of pointer in the implementation does not matter as C standard guarantees that any pointer to object type (not function pointer) can be converted to void *
and void *
can be converted to any type of pointer. How it is actually done is left to the implementation. So it is 100% safe as it is guaranteed by the C standard.
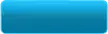