using this image as input
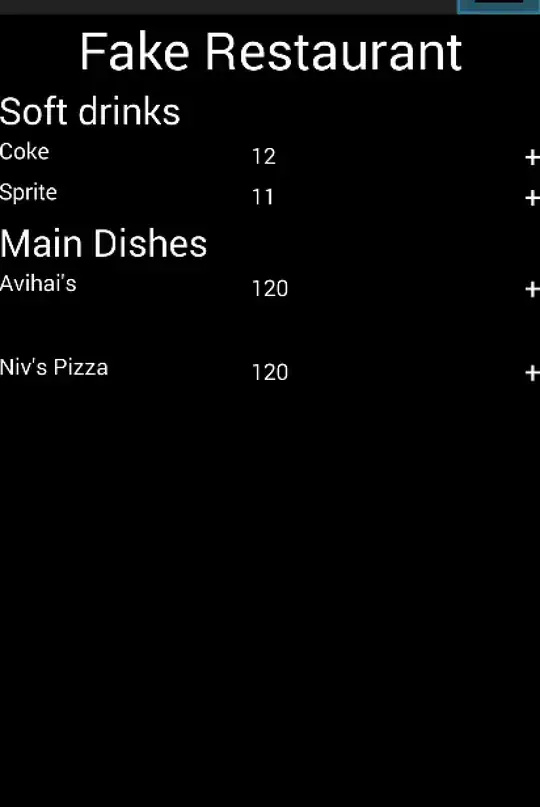
and this code:
#!/usr/bin/env python3
# -*- coding: utf-8 -*-
"""
Created on Wed Feb 3 10:41:37 2021
@author: Pietro
"""
import numpy as np
from PIL import Image
# import copy
image1 = Image.open('imgQ.tif', mode='r')
print('image size : ', image1.size)
print('image mode : ', image1.mode)
image2 = image1.convert('RGB')
print('image size : ', image2.size)
print('image mode : ', image2.mode)
def detect_edges (image: Image, threshold: float) -> Image:
image2_copy = image.copy()
image2_copy.show()
x, y = image2_copy.size
arrayz = (np.random.randint(0,1 ,(x , y))).astype(np.float)
print('arrayz shape : ',arrayz.shape)
print('arrayz dim :',arrayz.ndim, arrayz[0,:].shape, arrayz[:,0].shape)
print('arrayz type :',arrayz.dtype)
# for i in arrayz:
# print(i)
# print(arrayz)
pixels = image2_copy.load() # create the pixel map
for x in range(image2_copy.size[0]):
for y in range(image2_copy.size[1]):
r,g,b = pixels[x,y]
brightness = (r+b+g)/3
arrayz[x,y]=brightness
print(arrayz)
for x in range(image2_copy.size[0]):
for y in range(image2_copy.size[1]):
if abs(arrayz[x,y]-arrayz[x-1,y-1]) > threshold:
print('********************')
pixels[x,y] = (0,0,0)
else:
pixels[x,y] = (255,255,255)
print(pixels)
image2_copy.show()
image2_copy.save('imgQ_copy.tif')
# return new_image
imageM = detect_edges(image2, 100)
# imageM.show()
in python3 I get as result tis image:
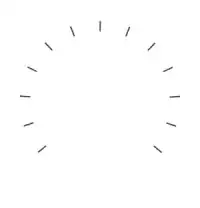
Not sure if thats what you were talking about,
and I belive there is something wrong in the approach used, if you zoom at the edges of the polygons the black edge is not present for some of the pixel, I think this is about the algorithm you were thinking, but could be due to my code as well, I am not versed on Images, hope someone better could shed some light on your question