I have looked at a couple of StackOverflow posts on how to get a data value by equating a child value that is few nodes deep.
In Firebase Database - How to perform a prefix wildcard query the structure of database is:

and the query ref.child("user_id").orderByChild("name").equalTo("objective-c")
works in pulling out child with particular uid.
In Firebase search by child value, the database structure is:
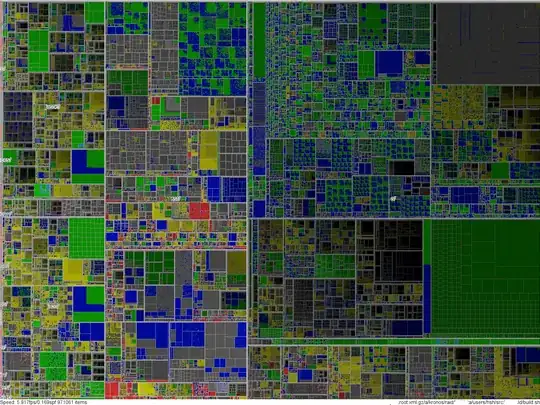
and the query ref.child('users').orderByChild('name').equalTo('John Doe')
is able to pull out the appropriate child.
In both these cases, the sorting and equating is on the grandchild. In my case, however, the database structure is a bit different. I want to index by a child property that is a few layers deep. The structure is:
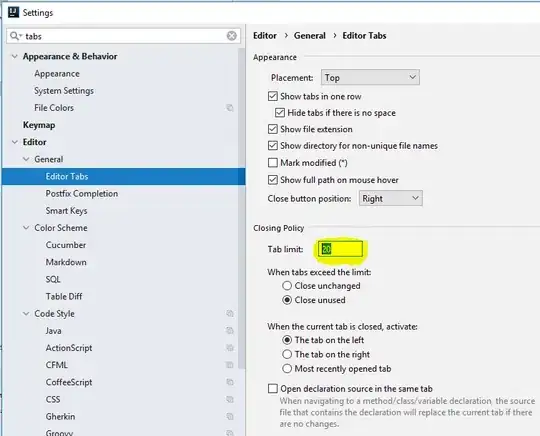
So I tried running the following query: authenticatedUsersReference.queryOrdered(byChild: fcmTokenKey).queryEqual(toValue: fcmToken).observeSingleEvent(of: .value)
, and my snapshot returned nil
. But when I ran authenticatedUsersReference.queryOrdered(byChild: fcmTokenKey).observe(.value)
, I saw that the snapshot values were ordered by the fcmTokens. authenticatedUsersReference
points to Database.database().reference().child("people/authenticated")
. I have also updated my rules to do indexing on the server side. As far as I can tell the concept should remain the same even if the depth changes, so what am I missing that is causing my query to return nil
?
"people": {
"users": {
"authenticated": {
"$userName": {
".indexOn": ["fcmToken"]
}
}
}
}