1- first you need the input - you need to get the getBearing() from the location object.
2- you need the correct output - you can do it in 2 ways -
a. if you use png icons , you can create an arrow for every 10 degrees, (36 arrows) and updated the marker with correct icon, if current bearing is different from the old bearing.
b. Better option but i don't know if this works: the marker of mapbox has options.rotation option. But in android, Mapbx moved to Mapbox annotation plugin, see also here
the SymbolManager has a function setIconRotationAlignment. so if you can use this class, i believe you can set the rotation of the icon by the bearing.
you can also see in this demo that one of the options is "set icon rotation to 45"
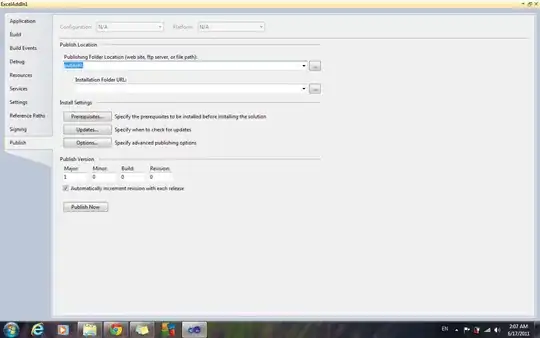
3- One more thing - you may want to get also input of getBearingAccuracyDegrees to know when you don't have a good enough Accuracy of the Bearing, and give indication to the user.