You seem happy with the help you've already gotten in the comments, but since you've tagged your question with [plotly]
, I thought you might be be interested in how to set up a table that lets you select any subset of unique values of time; [1, 2]
or the data as it is in your input dataframe.
Table 1 - Raw data

Table 2 - Subset with Time = 1
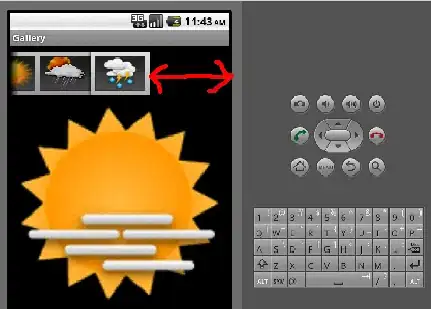
The comments in the code section should explain every step pretty clearly. Don't hesitate to let me know if anything should prove unclear.
Complete code:
import plotly.graph_objects as go
import pandas as pd
# input data
df = pd.DataFrame({'Time': {0: 1, 1: 1, 2: 1, 3: 2, 4: 2, 5: 2},
'Wavelength': {0: 100, 1: 101, 2: 102, 3: 100, 4: 101, 5: 101},
'Absorption': {0: 0.123,
1: 0.456,
2: 0.798,
3: 0.101,
4: 0.112,
5: 0.131}})
# plotly table setup
fig = go.Figure(go.Table(header=dict(values=df.columns.to_list()),
cells=dict(values=[df[col].to_list() for col in df.columns])
)
)
# set up buttons as list where the first element
# is an option for showing the raw data
buttons = [{'method': 'restyle',
'label': 'Raw data',
'args': [{'cells.values': [[df[col].to_list() for col in df.columns]],
'header.values': [df.columns.to_list()]},
],
}]
# create a dropdown option for each unique value of df['Time']
# which in this case is [1, 2]
# and extend the buttons list accordingly
for i, option in enumerate(df['Time'].unique()):
df_subset = (df[df['Time'] == option][['Wavelength', 'Absorption']])
buttons.extend([{'method': 'restyle',
'label': 'Time = ' + str(option),
'args': [{'cells.values': [[df_subset[col].to_list() for col in df_subset.columns]],
'header.values': [df_subset.columns.to_list()]},
],
},])
# configure updatemenu and add constructed buttons
updatemenus = [{'buttons': buttons,
'direction': 'down',
'showactive': True,}]
# update layout with buttons, and show the figure
fig.update_layout(updatemenus=updatemenus)
fig.show()