Beau Nouvelle solution is working, however, it's buggy and glitchy at least of me. Instead of using LazyVGrid
if we use HStack
with alignment: .top
It works better.
Here is the view
var body: some View {
HStack(alignment: .top) {
LazyVStack(spacing: 8) {
ForEach(splitArray[0]) {...}
}
LazyVStack(spacing: 8) {
ForEach(splitArray[1]) {...}
}
}
}
Here is the code to split the array
private var splitArray: [[Photo]] {
var result: [[Photo]] = []
var list1: [Photo] = []
var list2: [Photo] = []
photos.forEach { photo in
let index = photos.firstIndex {$0.id == photo.id }
if let index = index {
if index % 2 == 0 {
list1.append(photo)
} else {
list2.append(photo)
}
}
}
result.append(list1)
result.append(list2)
return result
}
I know this is not performance but so far only working solution I found.
Here is the full source code
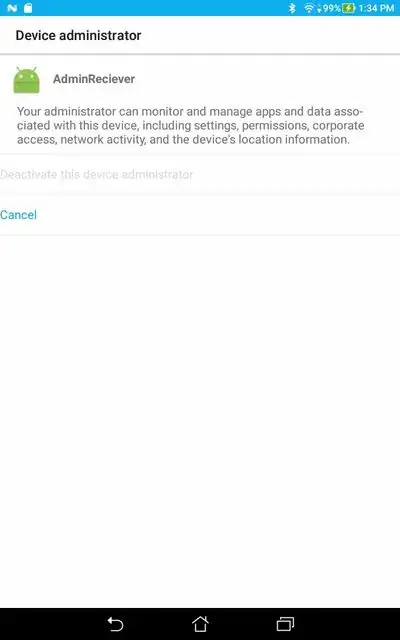