To get information about displays connected using WinUI 3, the simplest I can think of is to use
Nugget package: WindowsDisplayApi
Info here
It's a older package, however it works really well.
Once installed, you can do for example:
using System.Text.RegularExpressions;
using CommunityToolkit.Mvvm.ComponentModel;
using WindowsDisplayAPI;
namespace EasyDisplay.ViewModels;
public partial class DemoViewModel : ObservableObject
{
[ObservableProperty]
private string displayName = string.Empty;
[ObservableProperty]
private string friendly = string.Empty;
[ObservableProperty]
private string resolution = string.Empty;
[ObservableProperty]
private string position = string.Empty;
public DemoViewModel()
{
foreach (Display display in Display.GetDisplays())
{
DisplayName = display.DisplayName;
Friendly = GetFriendly(display.DisplayName);
Resolution = GetResolution(display);
Position = GetPosition(display);
}
}
private static string GetFriendly(string value)
{
return Regex.Replace(value, @"[^A-Za+Z0-9 ]", "");
}
private static string GetResolution(Display display)
{
return display.CurrentSetting.Resolution.Width.ToString() + " x " + display.CurrentSetting.Resolution.Height.ToString();
}
private static string GetPosition(Display display)
{
return display.CurrentSetting.Position.X.ToString() + " x " + display.CurrentSetting.Position.Y.ToString();
}
}
and more as per below:
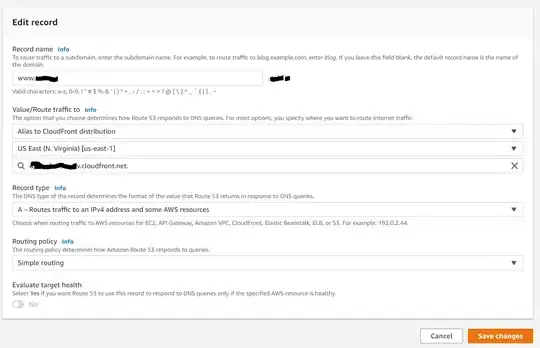
A second option using the Win32 APIs is available here, and may give you more functionalities.
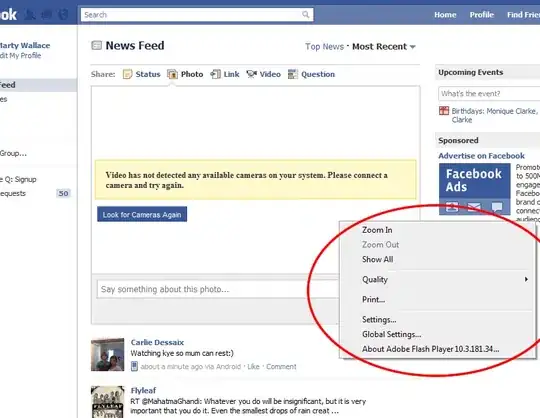