The following example uses seaborn's tips dataset. A histogram is created by grouping the total_bill
into bins. And then the bars are colored depending on the tips in each group.
import numpy as np
import pandas as pd
from matplotlib import pyplot as plt
import matplotlib.patches as mpatches
import seaborn as sns
sns.set_theme(style='white')
tips = sns.load_dataset('tips')
tips['bin'] = pd.cut(tips['total_bill'], 10) # histogram bin
grouped = tips.groupby('bin')
min_tip = tips['tip'].min()
max_tip = tips['tip'].max()
cmap = 'RdYlGn_r'
fig, ax = plt.subplots(figsize=(12, 4))
for bin, binned_df in grouped:
bin_height = len(binned_df)
binned_tips = np.sort(binned_df['tip']).reshape(-1, 1)
ax.imshow(binned_tips, cmap=cmap, vmin=min_tip, vmax=max_tip, extent=[bin.left, bin.right, 0, bin_height],
origin='lower', aspect='auto')
ax.add_patch(mpatches.Rectangle((bin.left, 0), bin.length, bin_height, fc='none', ec='k', lw=1))
ax.autoscale()
ax.set_ylim(0, 1.05 * ax.get_ylim()[1])
ax.set_xlabel('total bill')
ax.set_ylabel('frequency')
plt.colorbar(ax.images[0], ax=ax, label='tip')
plt.tight_layout()
plt.show()
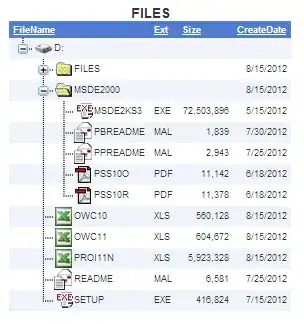
Here is how it looks with a banded colormap (cmap = plt.get_cmap('Spectral', 9)
):
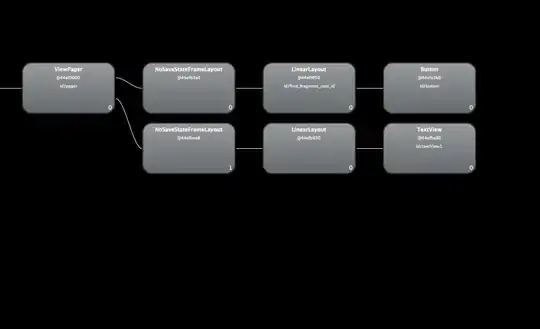
Here is another example using the 'mpg'
dataset, with a histogram over car weight and coloring via mile-per-gallon.
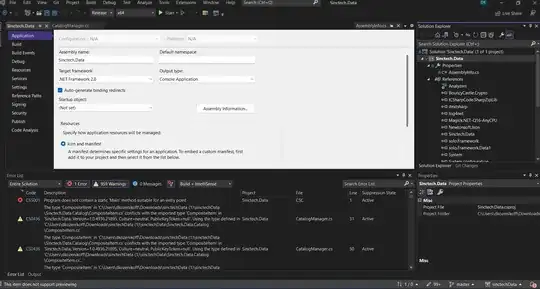