I am not quite sure if I understood your question right, but if you want to check if the input of the user is a substring of any word in your words vector, then Check if a string contains a string in C++ might be what you are looking for.
You could do the following to count the amount of times inputUser
was found in any word of your vector :
std::vector<std::string> words = { "Winter", "Summer", "Autmn", "inter" };
std::string inputOfUser = "int";
int countMatches = 0;
for (std::string word : words) {
if (word.find(inputOfUser) != std::string::npos) {
countMatches++;
}
}
std::cout << countMatches << std::endl;
In this example the output would be 2
EDIT (after question was edited)
As I think that I understood your problem now, I think this is probably what you are looking for:
#include <iostream>
#include <vector>
#include <string>
#include <algorithm>
std::vector<std::string> getSubStrings(char[], int n);
int main()
{
std::vector<std::string> words = { "winter", "summer", "autmn", "inter" };
std::string inputOfUser = "Winter";
std::vector<std::string> subStrings;
//transform the input to lower-case
std::transform(inputOfUser.begin(), inputOfUser.end(), inputOfUser.begin(), ::tolower);
//as getSubString requires a char[]
char* temp = &inputOfUser[0];
subStrings = getSubStrings(temp, inputOfUser.size());
// go through every substring
for (std::string subString : subStrings) {
std::cout << std::endl << "looking for substring: " << subString << std::endl;
// go through every word of your vector
for (std::string word : words) {
// does the word contain the substring?
if (word.find(subString) != std::string::npos){
// yes, it does
std::cout << "found: " << subString << " in word: " << word << std::endl;
}
//else: it does not
}
}
}
In this example, after getSubStrings(temp, inputOfUser.size())
is assigned to subStrings
, subStrings
would look like the following:
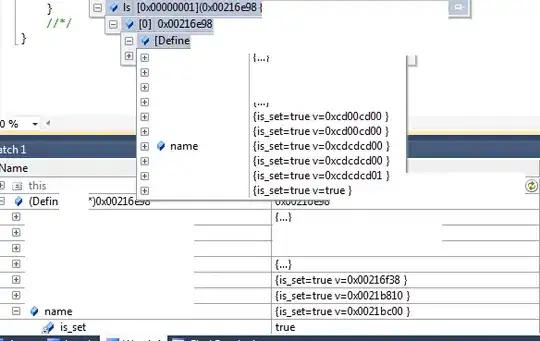
I took the getSubStrings()
method from https://www.geeksforgeeks.org/program-print-substrings-given-string/ and modified it a little bit, so it would fit for your case:
std::vector<std::string> getSubStrings(char str[], int n)
{
std::vector<std::string> subStrings;
// Pick starting point
for (int len = 1; len <= n; len++)
{
// Pick ending point
for (int i = 0; i <= n - len; i++)
{
std::string tempSub = "";
// take only the substrings that are greater than 2 in size
if (len > 2) {
// Add characters from current
// starting point to current ending
// point to tempSub
int j = i + len - 1;
for (int k = i; k <= j; k++) {
tempSub += str[k];
}
// push the resulted substring into subStrings
subStrings.push_back(tempSub);
}
}
}
return subStrings;
}
For the given example, the following would be the result ( remember, words looked like this std::vector<std::string> words = { "Winter", "Summer", "Autmn", "inter" };
and the input looked like this std::string inputOfUser = "Winter"
:
looking for substring: win
found: win in word: winter
looking for substring: int
found: int in word: winter
found: int in word: inter
looking for substring: nte
found: nte in word: winter
found: nte in word: inter
looking for substring: ter
found: ter in word: winter
found: ter in word: inter
looking for substring: wint
found: wint in word: winter
looking for substring: inte
found: inte in word: winter
found: inte in word: inter
looking for substring: nter
found: nter in word: winter
found: nter in word: inter
looking for substring: winte
found: winte in word: winter
looking for substring: inter
found: inter in word: winter
found: inter in word: inter
looking for substring: winter
found: winter in word: winter