Most importantly, dates have no idea what format they are in. It depends on the environment and output settings.
Configure your environment correctly
Specify the correct timezone in the manifest
Your project manifest must be set up for some time. It is important.
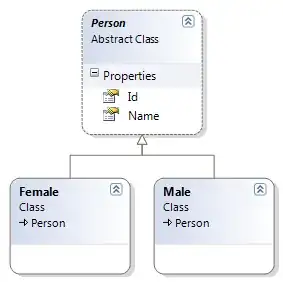
Customize the time display in Calendar as you need
You have to remember that dates are not what we see, they are more complex objects of information. What we see are strings from the dates.
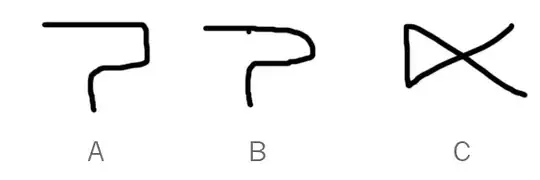
Let's code
The next code is correct
calendar
.createEvent(
"my title",
new Date('2021-01-27 8:00'),
new Date('2021-01-27 13:00')
);
because there is a correct signature for call new Date()
The next code is correct too
calendar
.createEvent(
"my title2",
new Date('2021-02-27 8:00'),
new Date('2021-02-27 8:00')
);
It creates an event with no duration. This is fine. This is logically expected.
To specify the interval you need specify noon time or 24 hour format:
calendar
.createEvent(
"my title2",
new Date('2021-02-27 8:00'),
new Date('2021-02-27 20:00')
);
or
calendar
.createEvent(
"my title2",
new Date('2021-02-27 8:00 AM'),
new Date('2021-02-27 8:00 PM')
);
Usually the duration is simply added to the start time. Like that
function createEvent() {
var calendar = CalendarApp.getDefaultCalendar();
const start = new Date('2021-02-27 8:00');
const end = new Date(start);
end.setHours(end.getHours() + 12);
calendar
.createEvent("my title2", start, end);
}
Yes, you can use your own formats for creating date:
function createEvent() {
var calendar = CalendarApp.getDefaultCalendar();
const start = newDate('27.02.2021 8:00');
const end = new Date(start);
end.setHours(end.getHours() + 12);
calendar
.createEvent("my title2", start, end);
}
function newDate(string){
const match = string.match(/(\d{1,2}).(\d{1,2}).(\d{4})\s+(\d{1,2}:\d{1,2})/);
if(!match) throw new Error('Invalid string');
return new Date(`${match[3]}-${match[2]}-${match[1]} ${match[4]}`);
}