tl;dr
To keep Map< Integer , String >
in an order sorted by key, use either of the two classes implementing the SortedMap
/NavigableMap
interfaces:
… or third-party implementations. Perhaps in Google Guava or Eclipse Collections (I’ve not checked).
If manipulating the map within a single thread, use the first, TreeMap
. If manipulating across threads, use the second, ConcurrentSkipListMap
.
For details, see the table below and the following discussion.
Details
Here is a graphic table I made showing the features of the ten Map
implementations bundled with Java 11.
The NavigableMap
interface is the successor to SortedMap
. The SortedMap
logically should be removed but cannot be as some 3rd-party map implementations may be using interface.
As you can see in this table, only two classes implement the SortedMap
/NavigableMap
interfaces:
Both of these keep keys in sorted order, either by their natural order (using compareTo
method of the Comparable
(https://docs.oracle.com/en/java/javase/11/docs/api/java.base/java/lang/Comparable.html) interface) or by a Comparator
implementation you pass. The difference between these two classes is that the second one, ConcurrentSkipListMap
, is thread-safe, highly concurrent.
See also the Iteration order column in the table below.
- The
LinkedHashMap
class returns its entries by the order in which they were originally inserted.
EnumMap
returns entries in the order by which the enum class of the key is defined. For example, a map of which employee is covering which day of the week (Map< DayOfWeek , Person >
) uses the DayOfWeek
enum class built into Java. That enum is defined with Monday first and Sunday last. So entries in an iterator will appear in that order.
The other six implementations make no promise about the order in which they report their entries.
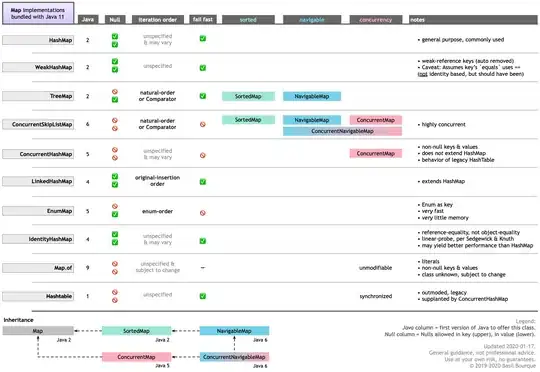