Good afternoon. I'm working on a quiz which contains four buttons. They contain a randomly selected line of text from a text file. The problem begins when I call 4 integers with the rand() function, which picks numbers from 1-5. Knowing that the rand() function can pick the same numbers, I code this:
if (random4 == random1 || random4 == random2 || random4 == random3) {
if (random4 == 5) {
while (random4 == random1 && random4 == random2 && random4 == random3 ||
random4 != 5) {
random4--;
}
return Button4Making();
} else {
while (random4 == random1 && random4 == random2 && random4 == random3) {
random4++;
}
return Button4Making();
}
}
But now my program works fine but crashes a lot of time. I'm assuming that I need to put a limit or something like that, but I don't know how to do it.
functions :
void game_frame::Button1Making() {
srand(time(NULL));
int bla = 0;
string rading;
ifstream object(
"C:\\Users\\Dzemail\\OneDrive\\Desktop\\database\\odgovori.dat");
while (bla != random1 && getline(object, rading)) {
++bla;
}
if (bla == random1) {
button1 = new wxButton(main_panel1, wxID_ANY, rading, wxPoint(10, 10),
wxSize(150, 50));
} else {
button1 = new wxButton(main_panel1, wxID_ANY, "Error", wxPoint(10, 10),
wxSize(150, 50));
}
}
void game_frame::Button2Making() {
srand(time(NULL));
int bla = 0;
if (random2 == random3 || random2 == random4 || random2 == random1) {
if (random2 == 5) {
while (random2 == random1 && random2 == random4 && random2 == random3) {
random2--;
}
return Button2Making();
} else {
while (random2 == random1 && random2 == random4 && random2 == random3 ||
random2 != 5) {
random2++;
}
return Button2Making();
}
} else {
string rading;
ifstream object(
"C:\\Users\\Dzemail\\OneDrive\\Desktop\\database\\odgovori.dat");
while (bla != random2 && getline(object, rading)) {
++bla;
}
if (bla == random2) {
button2 = new wxButton(main_panel1, wxID_ANY, rading, wxPoint(200, 10),
wxSize(150, 50));
} else {
button2 = new wxButton(main_panel1, wxID_ANY, "Error", wxPoint(200, 10),
wxSize(150, 50));
}
}
}
void game_frame::Button3Making() {
srand(time(NULL));
int bla = 0;
string rading;
ifstream object(
"C:\\Users\\Dzemail\\OneDrive\\Desktop\\database\\odgovori.dat");
while (bla != random3 && getline(object, rading)) {
++bla;
}
if (bla == random3) {
button3 = new wxButton(main_panel1, wxID_ANY, rading, wxPoint(400, 10),
wxSize(150, 50));
} else {
button3 = new wxButton(main_panel1, wxID_ANY, "Error", wxPoint(400, 10),
wxSize(150, 50));
}
}
void game_frame::Button4Making() {
srand(time(NULL));
int bla = 0;
if (random4 == random1 || random4 == random2 || random4 == random3) {
if (random4 == 5) {
while (random4 == random1 && random4 == random2 && random4 == random3 ||
random4 != 5) {
random4--;
}
return Button4Making();
} else {
while (random4 == random1 && random4 == random2 && random4 == random3) {
random4++;
}
return Button4Making();
}
} else {
string rading;
ifstream object(
"C:\\Users\\Dzemail\\OneDrive\\Desktop\\database\\odgovori.dat");
while (bla != random4 && getline(object, rading)) {
++bla;
}
if (bla == random4) {
button4 = new wxButton(main_panel1, wxID_ANY, rading, wxPoint(600, 10),
wxSize(150, 50));
} else {
button4 = new wxButton(main_panel1, wxID_ANY, "Error", wxPoint(600, 10),
wxSize(150, 50));
}
}
}
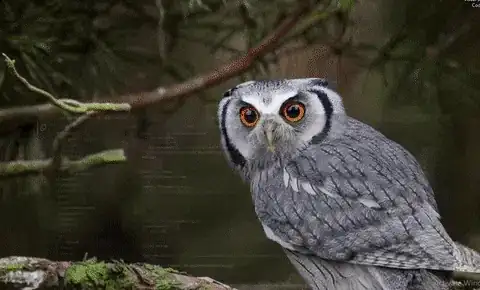