Your main issue is with this line
String dbfile = "C:/Users/user/Projects/AndroidStudioProjects/Bapp/Bapp_Projekt/app/src/main/assets/" + name;
You are basically trying to tell the device to look at your source code rather than to look for the asset file (the database) in the devices assets folder.
Android Studio (assuming you are using it) when it makes the package that is installed places the file in the assets folder not in c:/Users/user/Projects ....
In the tutorial (which doesn't use the assets file) but places the file on the SD card it uses:-
String dbfile = sdcard.getAbsolutePath() + File.separator+ "database" + File.separator + name;
However, as you have copied the file into the assets folder of the project then what you do is copy the file (the database) from the assets folder to a location (you cannot use the database from the assets folder directly as it is compressed).
Here's a quick quide that shows how the above can be done (note that step10 is for the tables/columns and would have to be changed accordingly)
how to launch app with SQLite darabase on Android Studio emulator?
An alternative would be to utilise SQLiteAssetHelper here's a guide that uses SQLiteAssetHelper .How to copy files from project assets folder to device data folder in android studio?
EXAMPLE based upon your code
Stage 1 - Create the external database
Using Navicat as the SQLite tool (my preferred tool)
The database was created with a single table namely item
The table has 2 columns namely item_name and item_type
4 rows were inserted 2 with an item_type of type1 and 2 with an item_type of type2 as per :-
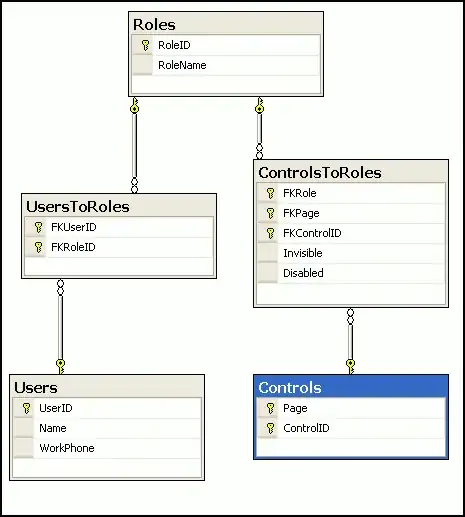
The database connection was closed and therefore saved and then the connection was reopened to confirm that the database is in fact populated, the connection was then closed again.
The existence of the database file was then confirmed to be at the appropriate location as per :-
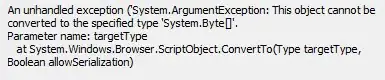
Stage 2 - Copy the database file into the assets folder
In this case the project has been called SO66390748 (the question #).
The assets folder was created in the Apps src/main folder as by default it doesn't exist and then the file copied into that folder noting that the size is as expected.
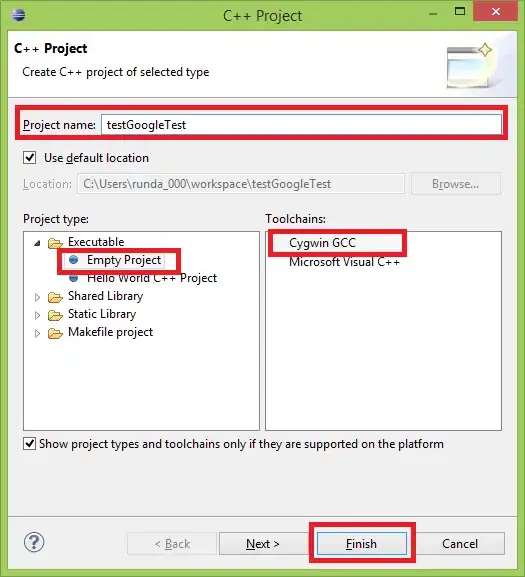
The project file structure was then tested in Android Studio to confirm the existence of the database in the asset folder :-
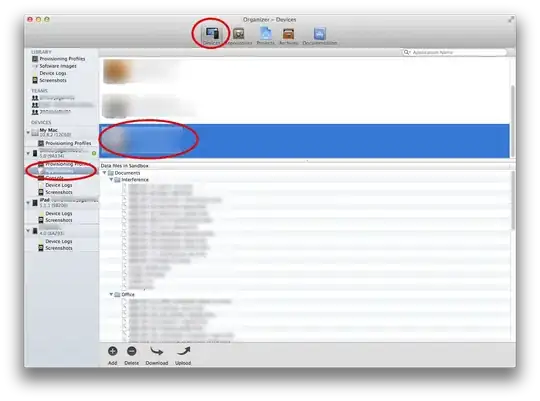
Stage 3 - Create the DatabaseHelper class as per
class DataBaseHelper extends SQLiteOpenHelper {
private static final String TAG = "DBHELPER";
public static final String DBNAME = "TestDB.db"; //<< Name of the database file in the assets folder
public static final int DBVERSION = 1;
public static final String TABLE_ITEM = "item";
public static final String ITEM_NAME = "item_name";
public static final String ITEM_TYPE = "item_type";
SQLiteDatabase mDB;
public DataBaseHelper(Context context) {
super(context,DBNAME,null,DBVERSION);
if (!ifDBExists(context)) {
if (!copyDBFromAssets(context)) {
throw new RuntimeException("Failed to Copy Database From Assets Folder");
}
}
mDB = this.getWritableDatabase();
}
@Override
public void onCreate(SQLiteDatabase sqLiteDatabase) {
// Do NOTHING in here as the database has been copied from the assets
// if it did not already exist
Log.d(TAG, "METHOD onCreate called");
}
@Override
public void onUpgrade(SQLiteDatabase sqLiteDatabase, int i, int i1) {
Log.d(TAG,"METHOD onUpgrade called");
}
public Cursor getDataDB_TableItemNamesByItemType(String itemType) {
SQLiteDatabase db = this.getWritableDatabase();
Cursor res = db.rawQuery("select * from " + TABLE_ITEM + " where "
+ ITEM_TYPE + " = '" + itemType+ "'", null);
Log.e("LogTag", "res.getCount(): " + res.getCount());
return res;
}
/*
Copies the database from the assets folder to the apps database folder (with logging)
note databases folder is typically data/data/the_package_name/database
however using getDatabasePath method gets the actual path (should it not be as above)
This method can be significantly reduced one happy that it works.
*/
private boolean copyDBFromAssets(Context context) {
Log.d("CPYDBINFO","Starting attemtpt to cop database from the assets file.");
String DBPATH = context.getDatabasePath(DBNAME).getPath();
InputStream is;
OutputStream os;
int buffer_size = 8192;
int length = buffer_size;
long bytes_read = 0;
long bytes_written = 0;
byte[] buffer = new byte[length];
try {
is = context.getAssets().open(DBNAME);
} catch (IOException e) {
Log.e("CPYDB FAIL - NO ASSET","Failed to open the Asset file " + DBNAME);
e.printStackTrace();
return false;
}
try {
os = new FileOutputStream(DBPATH);
} catch (IOException e) {
Log.e("CPYDB FAIL - OPENDB","Failed to open the Database File at " + DBPATH);
e.printStackTrace();
return false;
}
Log.d("CPYDBINFO","Initiating copy from asset file" + DBNAME + " to " + DBPATH);
while (length >= buffer_size) {
try {
length = is.read(buffer,0,buffer_size);
} catch (IOException e) {
Log.e("CPYDB FAIL - RD ASSET",
"Failed while reading in data from the Asset. " +
String.valueOf(bytes_read) +
" bytes read successfully."
);
e.printStackTrace();
return false;
}
bytes_read = bytes_read + length;
try {
os.write(buffer,0,buffer_size);
} catch (IOException e) {
Log.e("CPYDB FAIL - WR ASSET","failed while writing Database File " +
DBPATH +
". " +
String.valueOf(bytes_written) +
" bytes written successfully.");
e.printStackTrace();
return false;
}
bytes_written = bytes_written + length;
}
Log.d("CPYDBINFO",
"Read " + String.valueOf(bytes_read) + " bytes. " +
"Wrote " + String.valueOf(bytes_written) + " bytes."
);
try {
os.flush();
is.close();
os.close();
} catch (IOException e ) {
Log.e("CPYDB FAIL - FINALISING","Failed Finalising Database Copy. " +
String.valueOf(bytes_read) +
" bytes read." +
String.valueOf(bytes_written) +
" bytes written."
);
e.printStackTrace();
return false;
}
return true;
}
/*
Checks to see if the database exists if not will create the respective directory (database)
Creating the directory overcomes the NOT FOUND error
*/
private boolean ifDBExists(Context context) {
String dbparent = context.getDatabasePath(DBNAME).getParent();
File f = context.getDatabasePath(DBNAME);
if (!f.exists()) {
Log.d("NODB MKDIRS","Database file not found, making directories."); //<<<< remove before the App goes live.
File d = new File(dbparent);
d.mkdirs();
//return false;
}
return f.exists();
}
}
- As you can see your original
getDataDB_TableItemNamesByItemType
has been included unchanged.
- As per the comments (I'd suggest reading them) the above is a little long-winded BUT this enables you to see what is happening. Obviously remove the logging before distributing the App.
Stage 4 - Invoke the database helper and extract the data from the database
In this case the App's main activity is used to invoke.
The activity used is :-
public class MainActivity extends AppCompatActivity {
DataBaseHelper myDBHlpr;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// Get an instance of the DatabaseHelper class (copy will be done IF DB does not exist)
myDBHlpr = new DataBaseHelper(this);
// Get some data from the database using your method
Cursor csr = myDBHlpr.getDataDB_TableItemNamesByItemType("type2");
while(csr.moveToNext()){
Log.d("DB_ROWINFO",
"ITEM NAME is " + csr.getString(csr.getColumnIndex(DataBaseHelper.ITEM_NAME))
+ "ITEM TYPE is "
+ csr.getString((csr.getColumnIndex(DataBaseHelper.ITEM_TYPE))
)
);
}
// ========================================
// ALWAYS CLOSE CURSORS WHEN DONE WITH THEM
// ========================================
csr.close();
}
}
Stage 5 - The Results (the log)
The App if it exists is uninstalled then run and produces:-
03-06 09:32:52.759 5341-5341/a.a.so66390748 I/art: Rejecting re-init on previously-failed class java.lang.Class<androidx.core.view.ViewCompat$2>
03-06 09:32:52.787 5341-5341/a.a.so66390748 D/NODB MKDIRS: Database file not found, making directories.
03-06 09:32:52.787 5341-5341/a.a.so66390748 D/CPYDBINFO: Starting attemtpt to cop database from the assets file.
03-06 09:32:52.787 5341-5341/a.a.so66390748 D/CPYDBINFO: Initiating copy from asset fileTestDB.db to /data/user/0/a.a.so66390748/databases/TestDB.db
03-06 09:32:52.787 5341-5341/a.a.so66390748 D/CPYDBINFO: Read 8191 bytes. Wrote 8191 bytes.
03-06 09:32:52.805 5341-5341/a.a.so66390748 D/DBHELPER: METHOD onCreate called
03-06 09:32:52.811 5341-5341/a.a.so66390748 E/LogTag: res.getCount(): 2
03-06 09:32:52.811 5341-5341/a.a.so66390748 D/DB_ROWINFO: ITEM NAME is Item2ITEM TYPE is type2
03-06 09:32:52.811 5341-5341/a.a.so66390748 D/DB_ROWINFO: ITEM NAME is Item4ITEM TYPE is type2
03-06 09:32:52.822 5341-5355/a.a.so66390748 D/OpenGLRenderer: Use EGL_SWAP_BEHAVIOR_PRESERVED: true
The App is rerun a second time (not uninstalled) and the log is :-
03-06 09:35:37.876 5465-5465/a.a.so66390748 I/art: Rejecting re-init on previously-failed class java.lang.Class<androidx.core.view.ViewCompat$2>
03-06 09:35:37.908 5465-5465/a.a.so66390748 E/LogTag: res.getCount(): 2
03-06 09:35:37.908 5465-5465/a.a.so66390748 D/DB_ROWINFO: ITEM NAME is Item2ITEM TYPE is type2
03-06 09:35:37.908 5465-5465/a.a.so66390748 D/DB_ROWINFO: ITEM NAME is Item4ITEM TYPE is type2
03-06 09:35:37.956 5465-5498/a.a.so66390748 D/OpenGLRenderer: Use EGL_SWAP_BEHAVIOR_PRESERVED: true
i.e. The database, as it already exists and the database thus exists, doesn't copy the database but still extracts the data as expected.
Note the above has been written at my convenience so just Main activity and the database helper are used. You will obviously have to adapt the code accordingly
It is assumed that you followed the advice given in the comments and tried SELECT * FROM the_table_name
(i.e. no WHERE clause). I says this as the query you have used is case sensitive and if the argument passed to your getDataDB_TableItemNamesByItemType
method doesn't exactly match, then you would extract nothing. (e.g. passing Type2 instead of type2 shows 0 from the count)