I know I can just check in a Calendar or Date object, if it's
yesterday+1 ...
The java.util
date-time API and their formatting API, SimpleDateFormat
are outdated and error-prone. It is recommended to stop using them completely and switch to the modern date-time API.
But that is not considering the time, right? Because if the action is
done on 24.02. 7AM, then it would have to be 25.02. 7AM+ (24hrs) for
it to work?
The java.time API (the modern date-time API) provides you with LocalDateTime
to deal with local date and time (i.e. the date and time of a place and not requiring comparing it with the date and time of another place and hence not dealing with the timezone). However, when it comes to comparing it with the date and time of another place, not in the same timezone, you need ZonedDateTime
(to automatically adjust date & time object as per the DST) or OffsetDateTime
(to deal with ` fixed timezone offset) etc. Given below is an overview of java.time types:
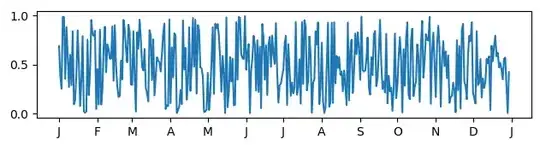
Demo:
import java.time.LocalDate;
import java.time.LocalDateTime;
import java.time.LocalTime;
public class Main {
public static void main(String args[]) {
LocalDate date = LocalDate.of(2020, 2, 23);
LocalTime time = LocalTime.of(7, 0);
LocalDateTime ldt = LocalDateTime.of(date, time);
System.out.println(ldt);
LocalDateTime afterTenHoursTwentyMinutes = ldt.plusHours(10).plusMinutes(20);
LocalDateTime tomorrow = ldt.plusDays(1);
LocalDateTime theDayAfterTomorrow = ldt.plusDays(2);
System.out.println(afterTenHoursTwentyMinutes);
System.out.println(tomorrow);
System.out.println(theDayAfterTomorrow);
if (!afterTenHoursTwentyMinutes.isAfter(theDayAfterTomorrow)) {
System.out.println("After 10 hours and 20 minutes, the date & time will not go past " + tomorrow);
} else {
System.out.println("After 10 hours and 20 minutes, the date & time will go past " + tomorrow);
}
}
}
Output:
2020-02-23T07:00
2020-02-23T17:20
2020-02-24T07:00
2020-02-25T07:00
After 10 hours and 20 minutes, the date & time will not go past 2020-02-24T07:00
Learn more about the modern date-time API from Trail: Date Time.