If one has access to Microsoft365's LET()
function, this could be done using a combination of functions:
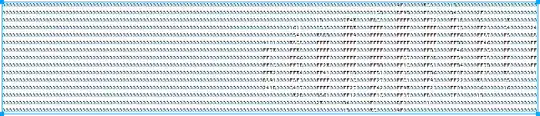
Formula in E1
:
=LET(X,SORT(TRIM(FILTERXML("<t><s>"&SUBSTITUTE(TEXTJOIN("#",,A1:C2),"#","</s><s>")&"</s></t>","//s[.!='']"))),INDEX(SORT(CHOOSE({1,2},UNIQUE(X),MMULT(--(UNIQUE(X)=TRANSPOSE(X)),SEQUENCE(COUNTA(X),,,0))),2,-1),{1;2;3;4;5},{1,2}))
Explanation:
LET()
allows the use of re-usable variables inside a formula. So in the above we assigned an array of values and named it "X". The array is pulled by:
=SORT(TRIM(FILTERXML("<t><s>"&SUBSTITUTE(TEXTJOIN("#",,A1:C2),"#","</s><s>")&"</s></t>","//s[.!='']")))
Where:
"<t><s>"&SUBSTITUTE(TEXTJOIN("#",,A1:C2),"#","</s><s>")&"</s></t>"
- Is used to create a valid XML-string and;
"//s[.!='']"
- A valid xpath to retrieve all non-empty strings.
TRIM()
will delete any leading and trailing spaces. SORT()
then sorts the array ascending.
If you want to know more about this mechanic to "split" a string into elements using FILTERXML()
, you can have a read here.
Now we have a variable, we can use it in the third parameter inside LET()
:
=INDEX(SORT(CHOOSE({1,2},UNIQUE(X),MMULT(--(UNIQUE(X)=TRANSPOSE(X)),SEQUENCE(COUNTA(X),,,0))),2,-1),{1,2,3,4,5},{1;2})
Using INDEX()
we can "slice" rows/columns from an array, where:
SORT(CHOOSE({1,2},UNIQUE(X),MMULT(--(UNIQUE(X)=TRANSPOSE(X)),SEQUENCE(COUNTA(X),,,0))),2,-1)
- A bit of a complicated construct, but the idea here is that CHOOSE({1,2}
allows for a 2D-array where the 1st column is filled with UNIQUE()
values from "X" and the 2nd is the count of each unique element in the total array. The count is done using MMULT()
where each unique element is counted against the TRANSPOSE()
'd "X". Then the 2d-array is sorted on the 2nd column.
With the above out of the way, we can simply use INDEX()
to retrieve the 5 rows we need (and both columns) using:
=INDEX(<TheAbove>,{1;2;3;4;5},{1,2})