If the main purpose is to find intersections, between equations, the symbolic math library sympy could be helpful:
from sympy import symbols, Eq, solve
x, y = symbols('x y')
eq1 = Eq(y, x)
eq2 = Eq(y, -x)
eq3 = Eq(y, -2 * x + 2)
print(solve([eq1, eq2]))
print(solve([eq1, eq3]))
print(solve([eq2, eq3]))
Output:
{x: 0, y: 0}
{x: 2/3, y: 2/3}
{x: 2, y: -2}
To find interpolated intersections between numpy arrays, you can use the find_roots()
function from this post:
from matplotlib import pyplot as plt
import numpy as np
def find_roots(x, y):
s = np.abs(np.diff(np.sign(y))).astype(bool)
return x[:-1][s] + np.diff(x)[s] / (np.abs(y[1:][s] / y[:-1][s]) + 1)
x1 = np.linspace(-1, 1, 100)
y1 = x1
y2 = -x1
y3 = -2 * x1 + 2
plt.plot(x1, y1, color='dodgerblue')
plt.plot(x1, y2, color='dodgerblue')
plt.plot(x1, y3, color='dodgerblue')
for ya, yb in [(y1, y2), (y1, y3), (y2, y3)]:
x0 = find_roots(x1, ya - yb)
y0 = np.interp(x0, x1, ya)
print(x0, y0)
plt.plot(x0, y0, marker='o', ls='', ms=4, color='crimson')
plt.show()
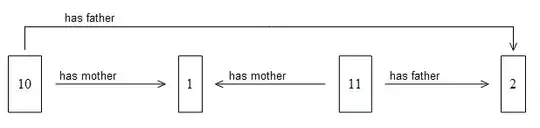
Output:
[0.] [0.]
[0.66666667] [0.66666667]
[] []
Zooming in on an intersection, and marking the points of the numpy arrays, you'll notice that the intersection usually doesn't coincide with a common point of the arrays. As such, an interpolation step is necessary.
