It is not completely clear to me what you want to achieve but this solution works for these test values and the second column:
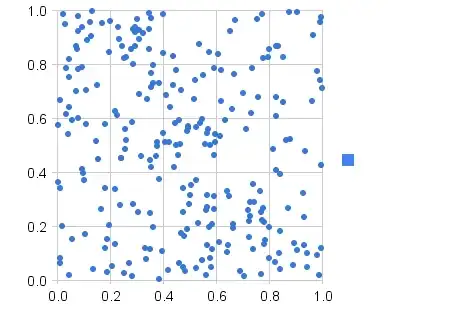
In your code I missed moving the selection in the sheet which I included here but it would be better to refer to cells in the sheet by the range rather than selecting cells anyway.
In the code I also included a method to print out the array after each step so you can check.
Option Explicit
Sub test()
Dim arr As Variant, _
mycell As Range, _
i, j, k As Long
arr = Range("F1:I8").Value
Debug.Print "Starting Point"
PrintArray arr
Do
For i = 2 To 2 'Just column 2 for now! normal: LBound(arr, 2) To UBound(arr, 2)
Range("A1").Select
For j = LBound(arr, 1) To UBound(arr, 1) 'Rows in that column
If arr(j, i) <> ActiveCell.Value Then
'shift down current column in array
For k = UBound(arr, 1) To j + 1 Step -1
arr(k, i) = arr(k - 1, i)
PrintArray arr
Next k
arr(j, i) = ActiveCell.Value
PrintArray arr
End If
ActiveCell.Offset(1, 0).Select
If ActiveCell = "" Then Exit For
Next j
Next i
Loop While ActiveCell <> ""
End Sub
Sub PrintArray(a As Variant)
Dim i, j As Long, _
line As String
For i = LBound(a, 1) To UBound(a, 1)
line = ""
For j = LBound(a, 2) To UBound(a, 2)
If a(i, j) = "" Then
line = line + "--- "
Else
line = line + Format(a(i, j), "000") + " "
End If
Next j
Debug.Print line
Next i
Debug.Print
End Sub
Output:
Starting Point
002 003 004 ---
004 006 008 ---
006 009 012 ---
008 012 016 ---
010 015 020 ---
012 018 024 ---
--- --- --- ---
--- --- --- ---
002 003 004 ---
004 006 008 ---
006 009 012 ---
008 012 016 ---
010 015 020 ---
012 018 024 ---
--- --- --- ---
--- --- --- ---
002 003 004 ---
004 006 008 ---
006 009 012 ---
008 012 016 ---
010 015 020 ---
012 018 024 ---
--- 018 --- ---
--- --- --- ---
002 003 004 ---
004 006 008 ---
006 009 012 ---
008 012 016 ---
010 015 020 ---
012 015 024 ---
--- 018 --- ---
--- --- --- ---
002 003 004 ---
004 006 008 ---
006 009 012 ---
008 012 016 ---
010 012 020 ---
012 015 024 ---
--- 018 --- ---
--- --- --- ---
002 003 004 ---
004 006 008 ---
006 009 012 ---
008 009 016 ---
010 012 020 ---
012 015 024 ---
--- 018 --- ---
--- --- --- ---
002 003 004 ---
004 006 008 ---
006 777 012 ---
008 009 016 ---
010 012 020 ---
012 015 024 ---
--- 018 --- ---
--- --- --- ---