Text Content from the Selected Item
If you are using a simple ComboBox
with ComboBoxItem
s defined in xaml, then you may only need this:
MessageBox.Show((combobox1.SelectedItem as ComboBoxItem).Content.ToString());
The SelectedItem
property gives you access to the instance of the object that is selected, depending on the rendering templates used and how or if you have bound the items to the combo the above may no work.
If you cannot guarantee that the item is the type you are expeceting I encourage you to use pattern matching in scenarios like this instead of direct casting. The resulting code becomes more extensible when you need to handle cases like null
(no selection) or when the SelectedItem is a data bound record (and so not a ComboBoxItem
)
if (combobox1.SelectedItem is ComboBoxItem cbItem)
MessageBox.Show($"{cbItem.Content}");
// example of an alternate expected type pattern:
//else if (combobox1.SelectedItem is MyWidget widget)
// MessageBox.Show(widget.Description);
else
MessageBox.Show($"{combobox1.SelectedItem}");
Selected Text in an editable ComboBox:
if you specifically want to access the text that is partially selected in the ComboBox, then according to this advice How to get ComboBox.SelectedText in WPF
You should be able to access the selected text by accessing the internal TextBox that is used in the control template for the combo box:
var textBox = (TextBox)combobox1.Template.FindName("PART_EditableTextBox", combobox1);
MessageBox.Show(textBox.SelectedText);
Background - TextBox selected text:
Have a read at this WPF tutorial: The TextBox Control
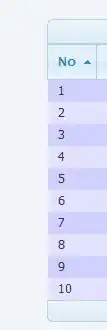
txtStatus.Text = "Selection starts at character #" + textBox.SelectionStart + Environment.NewLine;
txtStatus.Text += "Selection is " + textBox.SelectionLength + " character(s) long" + Environment.NewLine;
txtStatus.Text += "Selected text: '" + textBox.SelectedText + "'";
SelectionStart gives us the current cursor position or if there's a selection: Where it starts.
SelectionLength gives us the length of the current selection, if any. Otherwise it will just return 0.
SelectedText gives us the currently selected string if there's a selection. Otherwise an empty string is returned.