Do not try to control the game with an additional loop in the application loop. Use the application loop.
Add the variables is_jumping
adn jump_count
:
is_jumping = False
jump_count = 0
Use pygame.time.Clock
to control the frames per second and thus the game speed.
The method tick()
of a pygame.time.Clock
object, delays the game in that way, that every iteration of the loop consumes the same period of time. See pygame.time.Clock.tick()
:
This method should be called once per frame.
That means that the loop:
clock = pygame.time.Clock()
run = True
while run:
clock.tick(50)
runs 50 times per second.
Use the KEYDOWN
event instead of pygame.key.get_pressed()
to jump:
run = True
while run:
clock.tick(50)
for event in pygame.event.get():
if event.type == pygame.QUIT:
run = False
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_UP and not is_jumping:
is_jumping = True
jump_count = 30
pygame.key.get_pressed()
returns a list with the state of each key. If a key is held down, the state for the key is True
, otherwise False
. Use pygame.key.get_pressed()
to evaluate the current state of a button and get continuous movement.
The keyboard events (see pygame.event module) occur only once when the state of a key changes. The KEYDOWN
event occurs once every time a key is pressed. KEYUP
occurs once every time a key is released. Use the keyboard events for a single action.
Change the position of the player depending on is_jumping
adn jump_count
in the application loop:
while run:
# [...]
#movement code
if is_jumping and jump_count > 0:
if jump_count > 15:
player_y -= player_vel
jump_count -= 1
elif jump_count > 0:
player_y += player_vel
jump_count -= 1
is_jumping = jump_count > 0
Complete example:
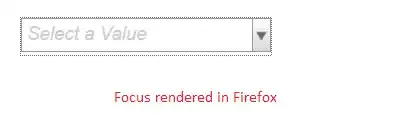
import pygame
pygame.init()
screen = pygame.display.set_mode((800, 600))
pygame.display.set_caption("Infinite Runner")
#defines the paremeters for the wall
wall_x = 800
wall_y = 300
wall_width = 20
wall_height = 20
score = 0
player_x = 400
player_y = 300
player_width = 40
player_height = 60
player_vel = 5
is_jumping = False
jump_count = 0
#where the main code is run
print(score)
clock = pygame.time.Clock()
run = True
while run:
clock.tick(50)
for event in pygame.event.get():
if event.type == pygame.QUIT:
run = False
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_UP and not is_jumping:
is_jumping = True
jump_count = 30
#movement code
if is_jumping and jump_count > 0:
if jump_count > 15:
player_y -= player_vel
jump_count -= 1
elif jump_count > 0:
player_y += player_vel
jump_count -= 1
is_jumping = jump_count > 0
#draws the player and the coin
screen.fill((0,0,0))
wall = pygame.draw.rect(screen, (244, 247, 30), (wall_x, wall_y, wall_width, wall_height))
player = pygame.draw.rect(screen, (255, 255, 255), (player_x, player_y, player_width, player_height))
pygame.display.update()
pygame.quit()